Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / ContainerVisual.cs / 1305600 / ContainerVisual.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: ContainerVisual.cs // // History: [....]: 05/28/2003 // Created it. //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Media.Effects; using System.Diagnostics; using System.Collections; using MS.Internal; using System.Resources; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// A ContainerVisual is a Container for other Visuals. /// public class ContainerVisual : Visual { ////// Ctor ContainerVisual. /// public ContainerVisual() { _children = new VisualCollection(this); } // ----------------------------------------------------------------------------------------- // Publicly re-exposed VisualTreeHelper interfaces. // ----------------------------------------------------------------------------------------- ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public VisualCollection Children { get { VerifyAPIReadOnly(); return _children; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public DependencyObject Parent { get { return base.VisualParent; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public Geometry Clip { get { return base.VisualClip; } set { base.VisualClip = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public double Opacity { get { return base.VisualOpacity; } set { base.VisualOpacity = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public Brush OpacityMask { get { return base.VisualOpacityMask; } set { base.VisualOpacityMask = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public CacheMode CacheMode { get { return base.VisualCacheMode; } set { base.VisualCacheMode = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// [Obsolete(MS.Internal.Media.VisualTreeUtils.BitmapEffectObsoleteMessage)] public BitmapEffect BitmapEffect { get { return base.VisualBitmapEffect; } set { base.VisualBitmapEffect = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// [Obsolete(MS.Internal.Media.VisualTreeUtils.BitmapEffectObsoleteMessage)] public BitmapEffectInput BitmapEffectInput { get { return base.VisualBitmapEffectInput; } set { base.VisualBitmapEffectInput = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public Effect Effect { get { return base.VisualEffect; } set { base.VisualEffect = value; } } ////// Gets or sets X- (vertical) guidelines on this Visual. /// [DefaultValue(null)] public DoubleCollection XSnappingGuidelines { get { return base.VisualXSnappingGuidelines; } set { base.VisualXSnappingGuidelines = value; } } ////// Gets or sets Y- (vertical) guidelines on this Visual. /// [DefaultValue(null)] public DoubleCollection YSnappingGuidelines { get { return base.VisualYSnappingGuidelines; } set { base.VisualYSnappingGuidelines = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// new public HitTestResult HitTest(Point point) { return base.HitTest(point); } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// new public void HitTest(HitTestFilterCallback filterCallback, HitTestResultCallback resultCallback, HitTestParameters hitTestParameters) { base.HitTest(filterCallback, resultCallback, hitTestParameters); } ////// VisualContentBounds returns the bounding box for the contents of this Visual. /// public Rect ContentBounds { get { return base.VisualContentBounds; } } ////// Gets or sets the Transform property. /// public Transform Transform { get { return base.VisualTransform; } set { base.VisualTransform = value; } } ////// Gets or sets the Offset property. /// public Vector Offset { get { return base.VisualOffset; } set { base.VisualOffset = value; } } ////// DescendantBounds returns the union of all of the content bounding /// boxes for all of the descendants of the current visual, but not including /// the contents of the current visual. /// public Rect DescendantBounds { get { return base.VisualDescendantBounds; } } // ------------------------------------------------------------------------------------------ // Protected methods // ----------------------------------------------------------------------------------------- ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// During this virtual call it is not valid to modify the Visual tree. /// protected sealed override Visual GetVisualChild(int index) { //VisualCollection does the range check for index return _children[index]; } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected sealed override int VisualChildrenCount { get { return _children.Count; } } // ------------------------------------------------------------------------------------------ // Private fields // ------------------------------------------------------------------------------------------ private readonly VisualCollection _children; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: ContainerVisual.cs // // History: [....]: 05/28/2003 // Created it. //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Media.Effects; using System.Diagnostics; using System.Collections; using MS.Internal; using System.Resources; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// A ContainerVisual is a Container for other Visuals. /// public class ContainerVisual : Visual { ////// Ctor ContainerVisual. /// public ContainerVisual() { _children = new VisualCollection(this); } // ----------------------------------------------------------------------------------------- // Publicly re-exposed VisualTreeHelper interfaces. // ----------------------------------------------------------------------------------------- ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public VisualCollection Children { get { VerifyAPIReadOnly(); return _children; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public DependencyObject Parent { get { return base.VisualParent; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public Geometry Clip { get { return base.VisualClip; } set { base.VisualClip = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public double Opacity { get { return base.VisualOpacity; } set { base.VisualOpacity = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public Brush OpacityMask { get { return base.VisualOpacityMask; } set { base.VisualOpacityMask = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public CacheMode CacheMode { get { return base.VisualCacheMode; } set { base.VisualCacheMode = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// [Obsolete(MS.Internal.Media.VisualTreeUtils.BitmapEffectObsoleteMessage)] public BitmapEffect BitmapEffect { get { return base.VisualBitmapEffect; } set { base.VisualBitmapEffect = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// [Obsolete(MS.Internal.Media.VisualTreeUtils.BitmapEffectObsoleteMessage)] public BitmapEffectInput BitmapEffectInput { get { return base.VisualBitmapEffectInput; } set { base.VisualBitmapEffectInput = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public Effect Effect { get { return base.VisualEffect; } set { base.VisualEffect = value; } } ////// Gets or sets X- (vertical) guidelines on this Visual. /// [DefaultValue(null)] public DoubleCollection XSnappingGuidelines { get { return base.VisualXSnappingGuidelines; } set { base.VisualXSnappingGuidelines = value; } } ////// Gets or sets Y- (vertical) guidelines on this Visual. /// [DefaultValue(null)] public DoubleCollection YSnappingGuidelines { get { return base.VisualYSnappingGuidelines; } set { base.VisualYSnappingGuidelines = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// new public HitTestResult HitTest(Point point) { return base.HitTest(point); } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// new public void HitTest(HitTestFilterCallback filterCallback, HitTestResultCallback resultCallback, HitTestParameters hitTestParameters) { base.HitTest(filterCallback, resultCallback, hitTestParameters); } ////// VisualContentBounds returns the bounding box for the contents of this Visual. /// public Rect ContentBounds { get { return base.VisualContentBounds; } } ////// Gets or sets the Transform property. /// public Transform Transform { get { return base.VisualTransform; } set { base.VisualTransform = value; } } ////// Gets or sets the Offset property. /// public Vector Offset { get { return base.VisualOffset; } set { base.VisualOffset = value; } } ////// DescendantBounds returns the union of all of the content bounding /// boxes for all of the descendants of the current visual, but not including /// the contents of the current visual. /// public Rect DescendantBounds { get { return base.VisualDescendantBounds; } } // ------------------------------------------------------------------------------------------ // Protected methods // ----------------------------------------------------------------------------------------- ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// During this virtual call it is not valid to modify the Visual tree. /// protected sealed override Visual GetVisualChild(int index) { //VisualCollection does the range check for index return _children[index]; } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected sealed override int VisualChildrenCount { get { return _children.Count; } } // ------------------------------------------------------------------------------------------ // Private fields // ------------------------------------------------------------------------------------------ private readonly VisualCollection _children; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
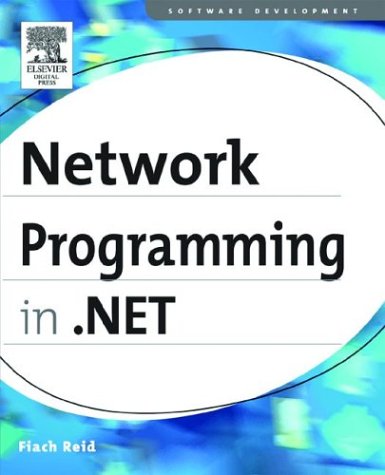
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerialPort.cs
- FactoryGenerator.cs
- HttpWrapper.cs
- WindowsClientCredential.cs
- _Win32.cs
- ComponentEvent.cs
- ModelTreeEnumerator.cs
- UnsafeNativeMethods.cs
- Image.cs
- Propagator.ExtentPlaceholderCreator.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- ButtonBaseAdapter.cs
- WindowsGraphics.cs
- SupportsEventValidationAttribute.cs
- JapaneseCalendar.cs
- PropertyGridCommands.cs
- DataRowView.cs
- SqlAliaser.cs
- DecimalFormatter.cs
- PropertyItemInternal.cs
- TextStore.cs
- ListDataHelper.cs
- ResumeStoryboard.cs
- LayoutEditorPart.cs
- UnSafeCharBuffer.cs
- SamlSubjectStatement.cs
- SyndicationContent.cs
- Line.cs
- columnmapkeybuilder.cs
- OdbcConnection.cs
- XPathSingletonIterator.cs
- ServiceBuildProvider.cs
- ModuleBuilder.cs
- TextTreeDeleteContentUndoUnit.cs
- GeometryConverter.cs
- WindowPattern.cs
- Win32SafeHandles.cs
- RTLAwareMessageBox.cs
- OracleSqlParser.cs
- HtmlInputHidden.cs
- EdmValidator.cs
- cache.cs
- TraceInternal.cs
- DataSourceCacheDurationConverter.cs
- EventSinkHelperWriter.cs
- VBIdentifierName.cs
- Vector3DAnimationUsingKeyFrames.cs
- httpserverutility.cs
- CryptoApi.cs
- TriState.cs
- CaseExpr.cs
- figurelengthconverter.cs
- SortQuery.cs
- BrushValueSerializer.cs
- UriParserTemplates.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- TemplateBindingExtensionConverter.cs
- Color.cs
- SpoolingTaskBase.cs
- ImmutableObjectAttribute.cs
- CodeTypeDelegate.cs
- DragStartedEventArgs.cs
- SafeFileHandle.cs
- WebPageTraceListener.cs
- EdmFunctions.cs
- CommandField.cs
- ServiceDurableInstance.cs
- ImportedNamespaceContextItem.cs
- DataTransferEventArgs.cs
- CustomCategoryAttribute.cs
- EntityClassGenerator.cs
- MaskInputRejectedEventArgs.cs
- AppDomainShutdownMonitor.cs
- ConstructorNeedsTagAttribute.cs
- ISCIIEncoding.cs
- DataBoundControl.cs
- ExpandedWrapper.cs
- RefExpr.cs
- MimeBasePart.cs
- SelectionChangedEventArgs.cs
- PropertyValidationContext.cs
- ElementAction.cs
- ComplexLine.cs
- Executor.cs
- XmlSchemaInferenceException.cs
- DataComponentGenerator.cs
- EntityUtil.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- SQLDecimal.cs
- CompensateDesigner.cs
- TextTreeExtractElementUndoUnit.cs
- DefinitionBase.cs
- MouseCaptureWithinProperty.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- ModelFactory.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- XamlTypeMapper.cs
- AssemblyEvidenceFactory.cs
- COM2ColorConverter.cs
- BufferedGraphicsContext.cs