Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Media / Animation / SetStoryboardSpeedRatio.cs / 1305600 / SetStoryboardSpeedRatio.cs
/****************************************************************************\ * * File: SetStoryboardSpeedRatio.cs * * This object includes a Storyboard reference. When triggered, the Storyboard * speed ratio is set to the given parameter. * * Copyright (C) by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System.ComponentModel; // DefaultValueAttribute using System.Diagnostics; // Debug.Assert namespace System.Windows.Media.Animation { ////// SetStoryboardSpeedRatio will set the speed for its Storyboard reference when /// it is triggered. /// public sealed class SetStoryboardSpeedRatio : ControllableStoryboardAction { ////// A speed ratio to use for this action. If it is never explicitly /// specified, it is 1.0. /// [DefaultValue(1.0)] public double SpeedRatio { get { return _speedRatio; } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "SetStoryboardSpeedRatio")); } _speedRatio = value; } } ////// Called when it's time to execute this storyboard action /// internal override void Invoke( FrameworkElement containingFE, FrameworkContentElement containingFCE, Storyboard storyboard ) { Debug.Assert( containingFE != null || containingFCE != null, "Caller of internal function failed to verify that we have a FE or FCE - we have neither." ); if( containingFE != null ) { storyboard.SetSpeedRatio(containingFE, SpeedRatio); } else { storyboard.SetSpeedRatio(containingFCE, SpeedRatio); } } double _speedRatio = 1.0; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
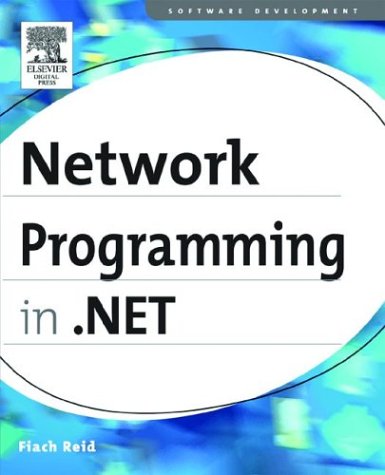
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinaryWriter.cs
- _FtpControlStream.cs
- OutOfMemoryException.cs
- MaskedTextBoxTextEditor.cs
- ObjectMemberMapping.cs
- SendMailErrorEventArgs.cs
- HttpGetProtocolReflector.cs
- RawStylusInputReport.cs
- ScriptComponentDescriptor.cs
- Selection.cs
- Latin1Encoding.cs
- XmlIlVisitor.cs
- List.cs
- VerificationException.cs
- LassoHelper.cs
- RadialGradientBrush.cs
- DragDeltaEventArgs.cs
- SqlColumnizer.cs
- EnumBuilder.cs
- RegexGroup.cs
- ColumnHeaderConverter.cs
- XPathScanner.cs
- VisualBasicExpressionConverter.cs
- RijndaelManaged.cs
- PageStatePersister.cs
- GridViewRowPresenter.cs
- CompressEmulationStream.cs
- LoginUtil.cs
- ObjectCloneHelper.cs
- NameNode.cs
- SubMenuStyleCollection.cs
- XmlSchemaRedefine.cs
- DesignerView.cs
- HtmlControlDesigner.cs
- BookmarkList.cs
- ChildrenQuery.cs
- ConditionalAttribute.cs
- LogLogRecordHeader.cs
- transactioncontext.cs
- WebPartMinimizeVerb.cs
- Rfc2898DeriveBytes.cs
- SourceFileInfo.cs
- ByteArrayHelperWithString.cs
- XamlWriter.cs
- SafeNativeMethods.cs
- RegexParser.cs
- Avt.cs
- BooleanKeyFrameCollection.cs
- propertyentry.cs
- OciEnlistContext.cs
- ErrorFormatterPage.cs
- InstanceLockTracking.cs
- ApplicationId.cs
- GlobalProxySelection.cs
- ExpressionNode.cs
- CategoryAttribute.cs
- DataPagerField.cs
- TimeSpan.cs
- SQLResource.cs
- FamilyMapCollection.cs
- BulletedListEventArgs.cs
- ContainerTracking.cs
- MobileUserControl.cs
- QueryExpr.cs
- DefaultProxySection.cs
- SqlMethodAttribute.cs
- ExpressionCopier.cs
- StringStorage.cs
- AttributeParameterInfo.cs
- CustomAttributeBuilder.cs
- TdsParserSessionPool.cs
- SlotInfo.cs
- DataGridRelationshipRow.cs
- MailBnfHelper.cs
- WebAdminConfigurationHelper.cs
- GestureRecognizer.cs
- CaseCqlBlock.cs
- SqlBuffer.cs
- InternalBase.cs
- Psha1DerivedKeyGenerator.cs
- ToolStripPanelCell.cs
- TargetException.cs
- SplineKeyFrames.cs
- PenThread.cs
- StorageMappingFragment.cs
- XslTransformFileEditor.cs
- MsmqOutputChannel.cs
- RemotingException.cs
- Logging.cs
- XPathChildIterator.cs
- CryptoHelper.cs
- Configuration.cs
- ProviderConnectionPointCollection.cs
- NamespaceDisplay.xaml.cs
- FormsAuthentication.cs
- CommandLibraryHelper.cs
- StoreAnnotationsMap.cs
- PagesChangedEventArgs.cs
- DesignSurface.cs
- ImmutableObjectAttribute.cs