Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / Design / Serialization / SerializationStore.cs / 1 / SerializationStore.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System.Collections; using System.ComponentModel; using System.IO; using System.Security.Permissions; ////// The SerializationStore class is an implementation-specific class that stores /// serialization data for the component serialization service. The /// service adds state to this serialization store. Once the store is /// closed it can be saved to a stream. A serialization store can be /// deserialized at a later date by the same type of serialization service. /// SerializationStore implements the IDisposable interface such that Dispose /// simply calls the Close method. Dispose is implemented as a private /// interface to avoid confusion. The IDisposable pattern is provided /// for languages that support a "using" syntax like C# and VB .NET. /// [HostProtection(SharedState = true)] public abstract class SerializationStore : IDisposable { ////// If there were errors generated during serialization or deserialization of the store, they will be /// added to this collection. /// public abstract ICollection Errors { get; } ////// The Close method closes this store and prevents any objects /// from being serialized into it. Once closed, the serialization store may be saved. /// public abstract void Close(); ////// The Save method saves the store to the given stream. If the store /// is open, Save will automatically close it for you. You /// can call save as many times as you wish to save the store /// to different streams. /// public abstract void Save(Stream stream); ////// Disposes this object by calling the Close method. /// void IDisposable.Dispose() {Dispose(true);} protected virtual void Dispose(bool disposing) { if(disposing) Close(); } } }
Link Menu
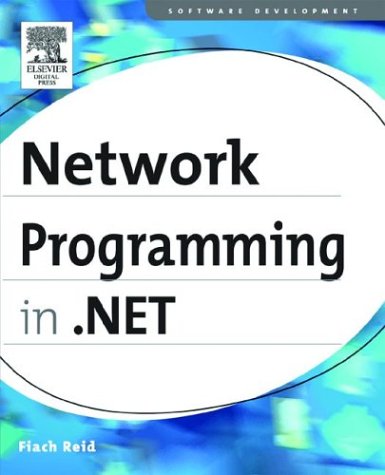
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RemoteWebConfigurationHost.cs
- EntityDesignerDataSourceView.cs
- FileSystemWatcher.cs
- FunctionDescription.cs
- MemberDescriptor.cs
- InvalidEnumArgumentException.cs
- SafeArrayTypeMismatchException.cs
- AppDomainFactory.cs
- __ComObject.cs
- ScrollEventArgs.cs
- QuaternionIndependentAnimationStorage.cs
- UdpContractFilterBehavior.cs
- RecognizedAudio.cs
- TypeTypeConverter.cs
- ParameterDataSourceExpression.cs
- PropertyCondition.cs
- HttpCookieCollection.cs
- DocumentPage.cs
- MsmqIntegrationReceiveParameters.cs
- CodeValidator.cs
- InnerItemCollectionView.cs
- HttpModuleAction.cs
- MissingMethodException.cs
- EntityDesignerBuildProvider.cs
- WorkflowServiceHost.cs
- RotateTransform3D.cs
- CapacityStreamGeometryContext.cs
- ValidationError.cs
- FixedSOMTable.cs
- ThousandthOfEmRealPoints.cs
- PostBackOptions.cs
- Context.cs
- wmiprovider.cs
- ProcessHostFactoryHelper.cs
- RoleService.cs
- KerberosReceiverSecurityToken.cs
- SystemColorTracker.cs
- EditingCommands.cs
- XhtmlBasicValidationSummaryAdapter.cs
- StateDesigner.Helpers.cs
- PageThemeParser.cs
- WebPartTransformerCollection.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- EncodedStreamFactory.cs
- DispatcherTimer.cs
- PerformanceCounterPermissionAttribute.cs
- GroupQuery.cs
- HMACSHA512.cs
- ProfileSection.cs
- ReadOnlyObservableCollection.cs
- StreamGeometry.cs
- SqlDataSourceStatusEventArgs.cs
- SocketPermission.cs
- ObjectListFieldCollection.cs
- ImageCollectionEditor.cs
- TextContainer.cs
- EventHandlingScope.cs
- XmlCharacterData.cs
- TextBox.cs
- SqlExpressionNullability.cs
- StylusLogic.cs
- AspNetCompatibilityRequirementsMode.cs
- DataTableClearEvent.cs
- AuthenticationModuleElement.cs
- FrameworkEventSource.cs
- CompilerTypeWithParams.cs
- ImageCodecInfoPrivate.cs
- ComboBoxRenderer.cs
- ObservableCollection.cs
- BindingExpression.cs
- CopyNamespacesAction.cs
- Timer.cs
- ServicePointManager.cs
- ColorDialog.cs
- DataTable.cs
- AppLevelCompilationSectionCache.cs
- BitmapEffectDrawing.cs
- WindowsPrincipal.cs
- EntityContainer.cs
- DbMetaDataCollectionNames.cs
- BaseProcessor.cs
- CacheAxisQuery.cs
- HtmlInputPassword.cs
- WindowsSlider.cs
- Fonts.cs
- ParameterCollection.cs
- JavascriptCallbackMessageInspector.cs
- RoleGroup.cs
- UniqueConstraint.cs
- SafeHandles.cs
- FontFamilyValueSerializer.cs
- OleDbConnectionInternal.cs
- RtType.cs
- GridView.cs
- BufferedGraphicsContext.cs
- QilStrConcat.cs
- ContextMarshalException.cs
- PointHitTestParameters.cs
- FileSystemEnumerable.cs
- TranslateTransform3D.cs