Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / SourceCollection.cs / 1 / SourceCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design{ using System; using System.Collections; using System.Diagnostics; using System.Design; ////// internal class SourceCollection : DataSourceCollectionBase, ICloneable { internal SourceCollection(DataSourceComponent collectionHost) : base(collectionHost) { } protected override Type ItemType { get { return typeof(Source); } } private DbSource MainSource { get{ DesignTable table = CollectionHost as DesignTable; return table.MainSource as DbSource; } } protected override INameService NameService { get { return SourceNameService.DefaultInstance; } } public int Add( Source s ) { return List.Add( s ); } public object Clone() { SourceCollection clone = new SourceCollection(null); foreach (Source s in this) { clone.Add((Source)s.Clone()); } return clone; } public bool Contains(Source s) { return List.Contains( s ); } bool DbSourceNameExist(DbSource dbSource, bool isFillName, string nameToBeChecked) { // Check both names even though a dbSource may only use one name at the time. // When a user change the DbSource's type to have two names, we then have a good starting name for her. // if (isFillName && StringUtil.EqualValue(nameToBeChecked, dbSource.GetMethodName, true)) { return true; } if (!isFillName && StringUtil.EqualValue(nameToBeChecked, dbSource.FillMethodName, true)) { return true; } foreach (DbSource s in this) { if (s != dbSource && s.NameExist(nameToBeChecked)){ return true; } } DbSource mainSource = MainSource; if (dbSource != mainSource && mainSource != null && mainSource.NameExist(nameToBeChecked)) { return true; } return false; } ////// /// ///internal override protected IDataSourceNamedObject FindObject(string name) { DbSource mainSource = MainSource; if (mainSource != null && mainSource.NameExist(name)) { return mainSource; } IEnumerator e = this.InnerList.GetEnumerator(); while (e.MoveNext()) { DbSource dbSource = e.Current as DbSource; if (dbSource != null) { if (dbSource.NameExist(name)) { return dbSource; } } else { IDataSourceNamedObject existing = (IDataSourceNamedObject)e.Current; if (StringUtil.EqualValue(existing.Name, name, false /*caseinsensitive*/)) { return existing; } } } return null; } public int IndexOf(Source s) { return List.IndexOf(s); } public void Remove(Source s) { List.Remove( s ); } #if not_needed_yet /// /// MainSource is not included in the sources collection, we fix the name validation for MainSource here. /// As MainSource name is fixed and not editable, we don't need to call this function to do validation /// /// ///internal void ValidateNameForMainSource(string mainSourceNameTobeCheck) { base.ValidateName(null, mainSourceNameTobeCheck); } #endif /// /// As MainSource is not included in the sources collection, we fix the name validation for sources here. /// /// ///private void ValidateNameWithMainSource(object dbSourceToCheck, string nameToCheck) { DbSource mainSource = MainSource; if (dbSourceToCheck != mainSource && mainSource != null) { if (mainSource.NameExist(nameToCheck)){ throw new NameValidationException(SR.GetString(SR.CM_NameExist, nameToCheck)); } } } /// /// /// internal protected override void ValidateName(IDataSourceNamedObject obj) { DbSource dbSource = obj as DbSource; if (dbSource != null) { if ((dbSource.GenerateMethods & GenerateMethodTypes.Get) == GenerateMethodTypes.Get) { this.NameService.ValidateName(dbSource.GetMethodName); } if ((dbSource.GenerateMethods & GenerateMethodTypes.Fill) == GenerateMethodTypes.Fill) { this.NameService.ValidateName(dbSource.FillMethodName); } } else { base.ValidateName(obj); } } ////// /// internal protected override void ValidateUniqueName(IDataSourceNamedObject obj, string proposedName) { Debug.Assert(!(obj is DbSource), "We should not call this function if it is a dbsource"); ValidateNameWithMainSource(obj, proposedName); base.ValidateUniqueName(obj, proposedName); } internal void ValidateUniqueDbSourceName(DbSource dbSource, string proposedName, bool isFillName) { if (this.DbSourceNameExist(dbSource, isFillName, proposedName)) { throw new NameValidationException(SR.GetString(SR.CM_NameExist, proposedName)); } this.NameService.ValidateName(proposedName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
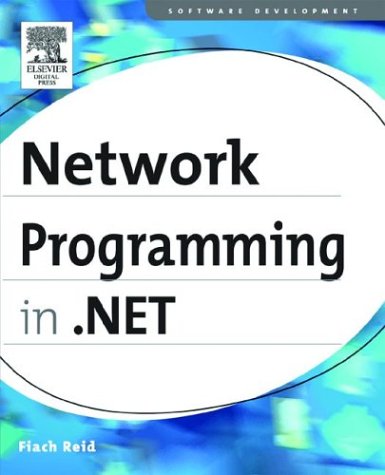
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeReference.cs
- TrustManagerPromptUI.cs
- WebEvents.cs
- Viewport3DAutomationPeer.cs
- Object.cs
- DataColumnMapping.cs
- TableLayoutRowStyleCollection.cs
- AttributeTable.cs
- PeerCredential.cs
- TableLayoutStyle.cs
- ThreadNeutralSemaphore.cs
- DelegateSerializationHolder.cs
- ViewStateException.cs
- ScriptReference.cs
- UrlMappingCollection.cs
- DocumentPageHost.cs
- FixedNode.cs
- TrackingQuery.cs
- SemaphoreSecurity.cs
- AtlasWeb.Designer.cs
- XmlQualifiedNameTest.cs
- EnterpriseServicesHelper.cs
- OpenTypeLayout.cs
- MailWriter.cs
- TabControlCancelEvent.cs
- SchemaObjectWriter.cs
- XpsViewerException.cs
- SequentialUshortCollection.cs
- ConnectionManagementSection.cs
- DataGridViewRowCollection.cs
- DrawToolTipEventArgs.cs
- DataBinding.cs
- SiteIdentityPermission.cs
- CompilationLock.cs
- FontInfo.cs
- TypeExtension.cs
- OpenTypeLayout.cs
- WebControlsSection.cs
- ArrayItemReference.cs
- SmtpDateTime.cs
- StrongNameHelpers.cs
- GlyphElement.cs
- BitmapFrame.cs
- TemplatedMailWebEventProvider.cs
- DataSpaceManager.cs
- SqlTriggerAttribute.cs
- PointAnimationClockResource.cs
- RankException.cs
- SqlErrorCollection.cs
- Pkcs7Signer.cs
- XmlSchemaImporter.cs
- FloaterParagraph.cs
- XDeferredAxisSource.cs
- Transform.cs
- ListItem.cs
- RtfFormatStack.cs
- DataGridViewAdvancedBorderStyle.cs
- DetailsView.cs
- DiscoveryVersion.cs
- Int64Converter.cs
- OperationValidationEventArgs.cs
- XmlSchemaChoice.cs
- RecognizedAudio.cs
- DataGridViewColumnEventArgs.cs
- HMACMD5.cs
- RuleValidation.cs
- SHA512Managed.cs
- XmlNullResolver.cs
- OpCodes.cs
- SamlAuthenticationClaimResource.cs
- EventArgs.cs
- StreamReader.cs
- SecurityTokenSerializer.cs
- StringFunctions.cs
- VirtualizingStackPanel.cs
- SqlCharStream.cs
- PtsCache.cs
- TextEffect.cs
- ImageCodecInfoPrivate.cs
- InvalidOperationException.cs
- ButtonStandardAdapter.cs
- BinaryObjectReader.cs
- ToolStripKeyboardHandlingService.cs
- ScriptComponentDescriptor.cs
- StringResourceManager.cs
- ExistsInCollection.cs
- NameValueConfigurationElement.cs
- ControlUtil.cs
- SoapMessage.cs
- BypassElement.cs
- odbcmetadatacolumnnames.cs
- RepeatBehavior.cs
- CodeMemberProperty.cs
- XmlWriterTraceListener.cs
- PathFigure.cs
- UserControlCodeDomTreeGenerator.cs
- PagerSettings.cs
- HtmlTernaryTree.cs
- DataControlHelper.cs
- MeshGeometry3D.cs