Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / TextBoxRenderer.cs / 1 / TextBoxRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TextBoxRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement TextBoxElement = VisualStyleElement.TextBox.TextEdit.Normal; //cannot instantiate private TextBoxRenderer() { } ////// This is a rendering class for the TextBox control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } private static void DrawBackground(Graphics g, Rectangle bounds, TextBoxState state) { visualStyleRenderer.DrawBackground(g, bounds); if (state != TextBoxState.Disabled) { Color windowColor = visualStyleRenderer.GetColor(ColorProperty.FillColor); if (windowColor != SystemColors.Window) { Rectangle fillRect = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); //then we need to re-fill the background. g.FillRectangle(new SolidBrush(SystemColors.Window), fillRect); } } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, TextBoxState state) { InitializeRenderer((int)state); DrawBackground(g, bounds, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, TextBoxState state) { DrawTextBox(g, bounds, textBoxText, font, TextFormatFlags.TextBoxControl, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, Rectangle textBounds, TextBoxState state) { DrawTextBox(g, bounds, textBoxText, font, textBounds, TextFormatFlags.TextBoxControl, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, TextFormatFlags flags, TextBoxState state) { InitializeRenderer((int)state); Rectangle textBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textBounds.Inflate(-2, -2); DrawTextBox(g, bounds, textBoxText, font, textBounds, flags, state); } ////// Renders a TextBox control. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, Rectangle textBounds, TextFormatFlags flags, TextBoxState state) { InitializeRenderer((int)state); DrawBackground(g, bounds, state); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, textBoxText, font, textBounds, textColor, flags); } private static void InitializeRenderer(int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Renders a TextBox control. /// ///
Link Menu
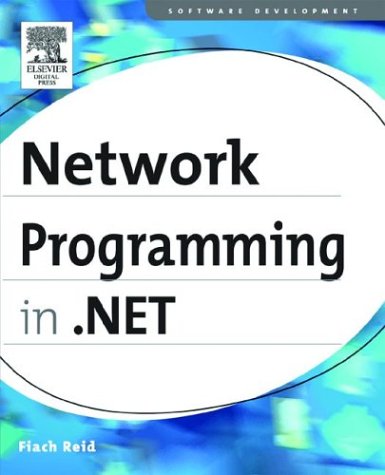
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataTable.cs
- FlowchartStart.xaml.cs
- FileDialog.cs
- ButtonFlatAdapter.cs
- PointCollection.cs
- ReadOnlyDictionary.cs
- TypeElement.cs
- CodeTypeOfExpression.cs
- ToolStripSplitStackLayout.cs
- SafeLibraryHandle.cs
- CustomPopupPlacement.cs
- BooleanConverter.cs
- TextElementEnumerator.cs
- GridViewRowPresenter.cs
- JsonReader.cs
- TransformerInfoCollection.cs
- CodeSubDirectory.cs
- SystemDiagnosticsSection.cs
- RequestSecurityToken.cs
- SplitterPanel.cs
- XmlSchemaObjectTable.cs
- PropertyInfoSet.cs
- ConfigurationValues.cs
- Geometry3D.cs
- TextReader.cs
- RequiredFieldValidator.cs
- _HelperAsyncResults.cs
- dataSvcMapFileLoader.cs
- SkinBuilder.cs
- ProcessStartInfo.cs
- TrackingDataItemValue.cs
- cookie.cs
- StreamingContext.cs
- SafeReversePInvokeHandle.cs
- MenuItemAutomationPeer.cs
- RuntimeEnvironment.cs
- SocketPermission.cs
- cookie.cs
- FileIOPermission.cs
- Single.cs
- SerializationHelper.cs
- PictureBox.cs
- StrongNameMembershipCondition.cs
- QilLoop.cs
- SyndicationFeedFormatter.cs
- oledbmetadatacollectionnames.cs
- LinqDataSourceContextEventArgs.cs
- ServiceObjectContainer.cs
- Stylesheet.cs
- TemplateControl.cs
- PresentationAppDomainManager.cs
- SqlDuplicator.cs
- HierarchicalDataSourceConverter.cs
- XPathParser.cs
- HttpCachePolicy.cs
- WebPartTransformerCollection.cs
- ConstraintManager.cs
- InvalidPipelineStoreException.cs
- CollectionViewProxy.cs
- SocketStream.cs
- RangeValuePatternIdentifiers.cs
- CompModHelpers.cs
- CmsUtils.cs
- Typography.cs
- HttpPostedFile.cs
- ListSortDescription.cs
- WorkflowOperationBehavior.cs
- Array.cs
- ListViewGroupItemCollection.cs
- NetDataContractSerializer.cs
- Pens.cs
- HtmlTable.cs
- XmlFormatWriterGenerator.cs
- SurrogateSelector.cs
- ByteStreamGeometryContext.cs
- Sql8ConformanceChecker.cs
- TextMessageEncoder.cs
- GroupBox.cs
- RadialGradientBrush.cs
- ImageKeyConverter.cs
- CompilerWrapper.cs
- Console.cs
- TemplatePropertyEntry.cs
- WebCategoryAttribute.cs
- WebServiceErrorEvent.cs
- CounterCreationData.cs
- Propagator.ExtentPlaceholderCreator.cs
- DataGridViewLayoutData.cs
- Binding.cs
- XmlSortKey.cs
- ClosableStream.cs
- UpdateRecord.cs
- BitmapScalingModeValidation.cs
- WebConfigurationFileMap.cs
- LambdaReference.cs
- SmtpNtlmAuthenticationModule.cs
- ErrorItem.cs
- SmiXetterAccessMap.cs
- AccessDataSourceView.cs
- DataGridPagerStyle.cs