Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / BooleanConverter.cs / 1 / BooleanConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public class BooleanConverter : TypeConverter { private static StandardValuesCollection values; ///Provides a type converter to convert /// Boolean objects to and from various other representations. ////// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ///Gets a value indicating whether this converter can /// convert an object in the given source type to a Boolean object using the /// specified context. ////// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { string text = ((string)value).Trim(); try { return Boolean.Parse(text); } catch (FormatException e) { throw new FormatException(SR.GetString(SR.ConvertInvalidPrimitive, (string)value, "Boolean"), e); } } return base.ConvertFrom(context, culture, value); } ///Converts the given value /// object to a Boolean object. ////// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (values == null) { values = new StandardValuesCollection(new object[] {true, false}); } return values; } ///Gets a collection of standard values /// for the Boolean data type. ////// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return true; } ///Gets a value indicating whether the list of standard values returned from /// ///is an exclusive list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } }Gets a value indicating whether this object supports a standard set of values /// that can be picked from a list. ///
Link Menu
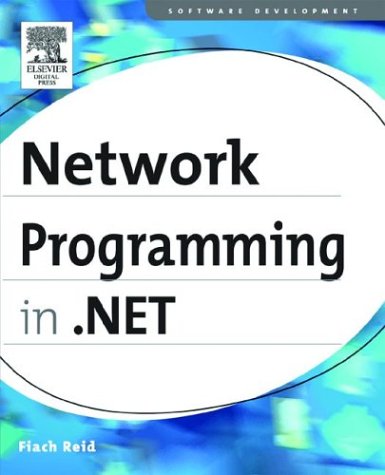
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripComboBox.cs
- UIAgentAsyncBeginRequest.cs
- StateRuntime.cs
- TextRenderer.cs
- StylusPointDescription.cs
- WeakReferenceEnumerator.cs
- DynamicRenderer.cs
- SchemaElement.cs
- Function.cs
- DataDocumentXPathNavigator.cs
- MulticastDelegate.cs
- XPathItem.cs
- BinaryObjectInfo.cs
- HyperLinkDesigner.cs
- ContentTextAutomationPeer.cs
- ViewValidator.cs
- LeafCellTreeNode.cs
- DataObjectFieldAttribute.cs
- NativeMethods.cs
- ApplicationInfo.cs
- ChildChangedEventArgs.cs
- PackageDigitalSignature.cs
- PrimaryKeyTypeConverter.cs
- KeyTimeConverter.cs
- NamespaceDecl.cs
- PenContext.cs
- ProtectedProviderSettings.cs
- LocalizeDesigner.cs
- InputScope.cs
- PenLineJoinValidation.cs
- ProfileSettings.cs
- ApplicationContext.cs
- VisualTarget.cs
- BitmapEffectCollection.cs
- BindingListCollectionView.cs
- TextFormatterHost.cs
- VerificationException.cs
- Queue.cs
- WebContentFormatHelper.cs
- SequenceDesigner.cs
- WebPartZoneCollection.cs
- PolygonHotSpot.cs
- CqlParserHelpers.cs
- NameTable.cs
- ServiceProviders.cs
- ArraySegment.cs
- TextContainer.cs
- AssemblyAttributes.cs
- XmlTypeAttribute.cs
- TheQuery.cs
- ObjectAnimationUsingKeyFrames.cs
- CollectionCodeDomSerializer.cs
- ModelUIElement3D.cs
- MimeParameters.cs
- UrlPath.cs
- LambdaCompiler.Address.cs
- BindingOperations.cs
- SchemaCollectionCompiler.cs
- sqlstateclientmanager.cs
- UInt64.cs
- XmlCharacterData.cs
- AudioFormatConverter.cs
- FontNamesConverter.cs
- PageDeviceFont.cs
- Tile.cs
- TextReader.cs
- HashLookup.cs
- GenerateTemporaryAssemblyTask.cs
- _NestedMultipleAsyncResult.cs
- WebScriptEnablingElement.cs
- FormsAuthenticationUser.cs
- Component.cs
- FixedSOMPageConstructor.cs
- DataGridViewRowConverter.cs
- webeventbuffer.cs
- WindowsGraphicsCacheManager.cs
- PaperSource.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- WebServiceEnumData.cs
- ClientUrlResolverWrapper.cs
- SqlNodeAnnotation.cs
- FrameworkPropertyMetadata.cs
- WindowsTitleBar.cs
- TrackingSection.cs
- BitmapMetadataBlob.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- StringArrayConverter.cs
- KeyedHashAlgorithm.cs
- TypeUtil.cs
- BaseTreeIterator.cs
- TreeNodeCollection.cs
- _ServiceNameStore.cs
- VScrollBar.cs
- UpdateCompiler.cs
- NavigationExpr.cs
- DecoderBestFitFallback.cs
- DiscoveryExceptionDictionary.cs
- RowUpdatedEventArgs.cs
- GuidelineCollection.cs
- MouseActionValueSerializer.cs