Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Documents / DocumentPaginator.cs / 1 / DocumentPaginator.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentPaginator.cs // // Description: This is the abstract base class for all paginating layouts. // It provides default implementations for the asynchronous // versions of GetPage and ComputePageCount. // // History: // 08/29/2005 : grzegorz - created. // //--------------------------------------------------------------------------- using System.ComponentModel; // AsyncCompletedEventArgs using System.Windows.Media; // Visual using MS.Internal.PresentationCore; // SR, SRID namespace System.Windows.Documents { ////// This is the abstract base class for all paginating layouts. It /// provides default implementations for the asynchronous versions of /// GetPage and ComputePageCount. /// public abstract class DocumentPaginator { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Retrieves the DocumentPage for the given page number. PageNumber /// is zero-based. /// /// Page number. ////// Returns DocumentPage.Missing if the given page does not exist. /// ////// Multiple requests for the same page number may return the same /// object (this is implementation specific). /// ////// Throws ArgumentOutOfRangeException if PageNumber is negative. /// public abstract DocumentPage GetPage(int pageNumber); ////// Async version of /// Page number. ////// /// Throws ArgumentOutOfRangeException if PageNumber is negative. /// public virtual void GetPageAsync(int pageNumber) { GetPageAsync(pageNumber, null); } ////// Async version of /// Page number. /// Unique identifier for the asynchronous task. ////// /// Throws ArgumentOutOfRangeException if PageNumber is negative. /// public virtual void GetPageAsync(int pageNumber, object userState) { DocumentPage page; // Page number cannot be negative. if (pageNumber < 0) { throw new ArgumentOutOfRangeException("pageNumber", SR.Get(SRID.PaginatorNegativePageNumber)); } page = GetPage(pageNumber); OnGetPageCompleted(new GetPageCompletedEventArgs(page, pageNumber, null, false, userState)); } ////// Computes the number of pages of content. IsPageCountValid will be /// True immediately after this is called. /// ////// If content is modified or PageSize is changed (or any other change /// that causes a repagination) after this method is called, /// IsPageCountValid will likely revert to False. /// public virtual void ComputePageCount() { // Force pagination of entire content. GetPage(int.MaxValue); } ////// Async version of public virtual void ComputePageCountAsync() { ComputePageCountAsync(null); } ////// /// Async version of /// Unique identifier for the asynchronous task. public virtual void ComputePageCountAsync(object userState) { ComputePageCount(); OnComputePageCountCompleted(new AsyncCompletedEventArgs(null, false, userState)); } ////// /// Cancels all asynchronous calls made with the given userState. /// If userState is NULL, all asynchronous calls are cancelled. /// /// Unique identifier for the asynchronous task. public virtual void CancelAsync(object userState) { } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Whether PageCount is currently valid. If False, then the value of /// PageCount is the number of pages that have currently been formatted. /// ////// This value may revert to False after being True, in cases where /// PageSize or content changes, forcing a repagination. /// public abstract bool IsPageCountValid { get; } ////// If IsPageCountValid is True, this value is the number of pages /// of content. If False, this is the number of pages that have /// currently been formatted. /// ////// Value may change depending upon changes in PageSize or content changes. /// public abstract int PageCount { get; } ////// The suggested size for formatting pages. /// ////// Note that the paginator may override the specified page size. Users /// should check DocumentPage.Size. /// public abstract Size PageSize { get; set; } ////// A pointer back to the element being paginated. /// public abstract IDocumentPaginatorSource Source { get; } #endregion Public Properties //-------------------------------------------------------------------- // // Public Events // //-------------------------------------------------------------------- #region Public Events ////// Fired when a GetPageAsync call has completed. /// public event GetPageCompletedEventHandler GetPageCompleted; ////// Fired when a ComputePageCountAsync call has completed. /// public event AsyncCompletedEventHandler ComputePageCountCompleted; ////// Fired when one of the properties of a DocumentPage changes. /// Affected pages must be re-fetched if currently used. /// ////// Existing DocumentPage objects may be destroyed when this /// event is fired. /// public event PagesChangedEventHandler PagesChanged; #endregion Public Events //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the GetPageCompleted event. protected virtual void OnGetPageCompleted(GetPageCompletedEventArgs e) { if (this.GetPageCompleted != null) { this.GetPageCompleted(this, e); } } ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the ComputePageCountCompleted event. protected virtual void OnComputePageCountCompleted(AsyncCompletedEventArgs e) { if (this.ComputePageCountCompleted != null) { this.ComputePageCountCompleted(this, e); } } ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the PagesChanged event. protected virtual void OnPagesChanged(PagesChangedEventArgs e) { if (this.PagesChanged != null) { this.PagesChanged(this, e); } } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentPaginator.cs // // Description: This is the abstract base class for all paginating layouts. // It provides default implementations for the asynchronous // versions of GetPage and ComputePageCount. // // History: // 08/29/2005 : grzegorz - created. // //--------------------------------------------------------------------------- using System.ComponentModel; // AsyncCompletedEventArgs using System.Windows.Media; // Visual using MS.Internal.PresentationCore; // SR, SRID namespace System.Windows.Documents { ////// This is the abstract base class for all paginating layouts. It /// provides default implementations for the asynchronous versions of /// GetPage and ComputePageCount. /// public abstract class DocumentPaginator { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Retrieves the DocumentPage for the given page number. PageNumber /// is zero-based. /// /// Page number. ////// Returns DocumentPage.Missing if the given page does not exist. /// ////// Multiple requests for the same page number may return the same /// object (this is implementation specific). /// ////// Throws ArgumentOutOfRangeException if PageNumber is negative. /// public abstract DocumentPage GetPage(int pageNumber); ////// Async version of /// Page number. ////// /// Throws ArgumentOutOfRangeException if PageNumber is negative. /// public virtual void GetPageAsync(int pageNumber) { GetPageAsync(pageNumber, null); } ////// Async version of /// Page number. /// Unique identifier for the asynchronous task. ////// /// Throws ArgumentOutOfRangeException if PageNumber is negative. /// public virtual void GetPageAsync(int pageNumber, object userState) { DocumentPage page; // Page number cannot be negative. if (pageNumber < 0) { throw new ArgumentOutOfRangeException("pageNumber", SR.Get(SRID.PaginatorNegativePageNumber)); } page = GetPage(pageNumber); OnGetPageCompleted(new GetPageCompletedEventArgs(page, pageNumber, null, false, userState)); } ////// Computes the number of pages of content. IsPageCountValid will be /// True immediately after this is called. /// ////// If content is modified or PageSize is changed (or any other change /// that causes a repagination) after this method is called, /// IsPageCountValid will likely revert to False. /// public virtual void ComputePageCount() { // Force pagination of entire content. GetPage(int.MaxValue); } ////// Async version of public virtual void ComputePageCountAsync() { ComputePageCountAsync(null); } ////// /// Async version of /// Unique identifier for the asynchronous task. public virtual void ComputePageCountAsync(object userState) { ComputePageCount(); OnComputePageCountCompleted(new AsyncCompletedEventArgs(null, false, userState)); } ////// /// Cancels all asynchronous calls made with the given userState. /// If userState is NULL, all asynchronous calls are cancelled. /// /// Unique identifier for the asynchronous task. public virtual void CancelAsync(object userState) { } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Whether PageCount is currently valid. If False, then the value of /// PageCount is the number of pages that have currently been formatted. /// ////// This value may revert to False after being True, in cases where /// PageSize or content changes, forcing a repagination. /// public abstract bool IsPageCountValid { get; } ////// If IsPageCountValid is True, this value is the number of pages /// of content. If False, this is the number of pages that have /// currently been formatted. /// ////// Value may change depending upon changes in PageSize or content changes. /// public abstract int PageCount { get; } ////// The suggested size for formatting pages. /// ////// Note that the paginator may override the specified page size. Users /// should check DocumentPage.Size. /// public abstract Size PageSize { get; set; } ////// A pointer back to the element being paginated. /// public abstract IDocumentPaginatorSource Source { get; } #endregion Public Properties //-------------------------------------------------------------------- // // Public Events // //-------------------------------------------------------------------- #region Public Events ////// Fired when a GetPageAsync call has completed. /// public event GetPageCompletedEventHandler GetPageCompleted; ////// Fired when a ComputePageCountAsync call has completed. /// public event AsyncCompletedEventHandler ComputePageCountCompleted; ////// Fired when one of the properties of a DocumentPage changes. /// Affected pages must be re-fetched if currently used. /// ////// Existing DocumentPage objects may be destroyed when this /// event is fired. /// public event PagesChangedEventHandler PagesChanged; #endregion Public Events //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the GetPageCompleted event. protected virtual void OnGetPageCompleted(GetPageCompletedEventArgs e) { if (this.GetPageCompleted != null) { this.GetPageCompleted(this, e); } } ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the ComputePageCountCompleted event. protected virtual void OnComputePageCountCompleted(AsyncCompletedEventArgs e) { if (this.ComputePageCountCompleted != null) { this.ComputePageCountCompleted(this, e); } } ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the PagesChanged event. protected virtual void OnPagesChanged(PagesChangedEventArgs e) { if (this.PagesChanged != null) { this.PagesChanged(this, e); } } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
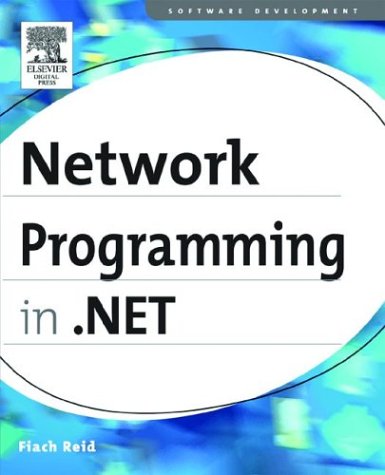
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HandleRef.cs
- DesignerLoader.cs
- NotifyInputEventArgs.cs
- JsonQueryStringConverter.cs
- x509utils.cs
- AuthorizationSection.cs
- TypeInfo.cs
- WindowsToolbarAsMenu.cs
- FrameworkTemplate.cs
- ExtendedTransformFactory.cs
- Atom10FormatterFactory.cs
- Label.cs
- RoleBoolean.cs
- WindowInteropHelper.cs
- DatagridviewDisplayedBandsData.cs
- ObservableDictionary.cs
- XmlSerializableServices.cs
- MatrixCamera.cs
- WeakHashtable.cs
- BadImageFormatException.cs
- VSWCFServiceContractGenerator.cs
- CircleHotSpot.cs
- GuidTagList.cs
- ChangeDirector.cs
- FilteredXmlReader.cs
- MetadataFile.cs
- EntityViewGenerationConstants.cs
- EntityDataSourceState.cs
- CodeCastExpression.cs
- BitmapMetadataEnumerator.cs
- MDIClient.cs
- BaseInfoTable.cs
- ControlIdConverter.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- PageCodeDomTreeGenerator.cs
- BuildManagerHost.cs
- sitestring.cs
- ByteConverter.cs
- WindowsAuthenticationModule.cs
- Predicate.cs
- DataGridBoolColumn.cs
- ResourceReader.cs
- UInt64Converter.cs
- ScriptManager.cs
- WorkflowEventArgs.cs
- Stylesheet.cs
- PathSegment.cs
- FixedSOMLineCollection.cs
- AssemblyBuilderData.cs
- GrammarBuilder.cs
- DoubleKeyFrameCollection.cs
- cookiecollection.cs
- ObjectListItem.cs
- DataMemberAttribute.cs
- DrawingGroup.cs
- SoapElementAttribute.cs
- ZoneButton.cs
- Int32Storage.cs
- CodeBlockBuilder.cs
- StrokeNodeOperations2.cs
- ReadOnlyTernaryTree.cs
- QilXmlReader.cs
- PreservationFileReader.cs
- CookielessHelper.cs
- TableItemPattern.cs
- TypeValidationEventArgs.cs
- oledbmetadatacollectionnames.cs
- SessionStateModule.cs
- ExtenderProviderService.cs
- GuidelineSet.cs
- ISAPIWorkerRequest.cs
- ObjectReaderCompiler.cs
- ProgressPage.cs
- CachedCompositeFamily.cs
- SqlAggregateChecker.cs
- CodeCommentStatement.cs
- IDispatchConstantAttribute.cs
- StyleTypedPropertyAttribute.cs
- CallbackHandler.cs
- ApplicationTrust.cs
- InvalidWMPVersionException.cs
- MailAddress.cs
- DataGridViewToolTip.cs
- DefaultHttpHandler.cs
- XmlNamespaceMappingCollection.cs
- Select.cs
- GuidTagList.cs
- LinqDataSourceDeleteEventArgs.cs
- SmtpReplyReaderFactory.cs
- ConfigurationLockCollection.cs
- SafeSecurityHandles.cs
- GridItemProviderWrapper.cs
- StringAttributeCollection.cs
- NavigationCommands.cs
- GradientBrush.cs
- SecurityException.cs
- SByteStorage.cs
- safex509handles.cs
- QilTargetType.cs
- TextContainer.cs