Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / ComboBoxRenderer.cs / 1 / ComboBoxRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class ComboBoxRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement ComboBoxElement = VisualStyleElement.ComboBox.DropDownButton.Normal; private static readonly VisualStyleElement TextBoxElement = VisualStyleElement.TextBox.TextEdit.Normal; //cannot instantiate private ComboBoxRenderer() { } ////// This is a rendering class for the ComboBox control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } private static void DrawBackground(Graphics g, Rectangle bounds, ComboBoxState state) { visualStyleRenderer.DrawBackground(g, bounds); //for disabled comboboxes, comctl does not use the window backcolor, so // we don't refill here in that case. if (state != ComboBoxState.Disabled) { Color windowColor = visualStyleRenderer.GetColor(ColorProperty.FillColor); if (windowColor != SystemColors.Window) { Rectangle fillRect = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); fillRect.Inflate(-2, -2); //then we need to re-fill the background. g.FillRectangle(SystemBrushes.Window, fillRect); } } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, ComboBoxState state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } DrawBackground(g, bounds, state); } ////// Renders the textbox part of a ComboBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string comboBoxText, Font font, ComboBoxState state) { DrawTextBox(g, bounds, comboBoxText, font, TextFormatFlags.TextBoxControl, state); } ////// Renders the textbox part of a ComboBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string comboBoxText, Font font, Rectangle textBounds, ComboBoxState state) { DrawTextBox(g, bounds, comboBoxText, font, textBounds, TextFormatFlags.TextBoxControl, state); } ////// Renders the textbox part of a ComboBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string comboBoxText, Font font, TextFormatFlags flags, ComboBoxState state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } Rectangle textBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textBounds.Inflate(-2,-2); DrawTextBox(g, bounds, comboBoxText, font, textBounds, flags, state); } ////// Renders the textbox part of a ComboBox control. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, string comboBoxText, Font font, Rectangle textBounds, TextFormatFlags flags, ComboBoxState state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } DrawBackground(g, bounds, state); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, comboBoxText, font, textBounds, textColor, flags); } ////// Renders the textbox part of a ComboBox control. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawDropDownButton(Graphics g, Rectangle bounds, ComboBoxState state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(ComboBoxElement.ClassName, ComboBoxElement.Part, (int)state); } else { visualStyleRenderer.SetParameters(ComboBoxElement.ClassName, ComboBoxElement.Part, (int)state); } visualStyleRenderer.DrawBackground(g, bounds); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Renders a ComboBox drop-down button. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class ComboBoxRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement ComboBoxElement = VisualStyleElement.ComboBox.DropDownButton.Normal; private static readonly VisualStyleElement TextBoxElement = VisualStyleElement.TextBox.TextEdit.Normal; //cannot instantiate private ComboBoxRenderer() { } ////// This is a rendering class for the ComboBox control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } private static void DrawBackground(Graphics g, Rectangle bounds, ComboBoxState state) { visualStyleRenderer.DrawBackground(g, bounds); //for disabled comboboxes, comctl does not use the window backcolor, so // we don't refill here in that case. if (state != ComboBoxState.Disabled) { Color windowColor = visualStyleRenderer.GetColor(ColorProperty.FillColor); if (windowColor != SystemColors.Window) { Rectangle fillRect = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); fillRect.Inflate(-2, -2); //then we need to re-fill the background. g.FillRectangle(SystemBrushes.Window, fillRect); } } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, ComboBoxState state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } DrawBackground(g, bounds, state); } ////// Renders the textbox part of a ComboBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string comboBoxText, Font font, ComboBoxState state) { DrawTextBox(g, bounds, comboBoxText, font, TextFormatFlags.TextBoxControl, state); } ////// Renders the textbox part of a ComboBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string comboBoxText, Font font, Rectangle textBounds, ComboBoxState state) { DrawTextBox(g, bounds, comboBoxText, font, textBounds, TextFormatFlags.TextBoxControl, state); } ////// Renders the textbox part of a ComboBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string comboBoxText, Font font, TextFormatFlags flags, ComboBoxState state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } Rectangle textBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textBounds.Inflate(-2,-2); DrawTextBox(g, bounds, comboBoxText, font, textBounds, flags, state); } ////// Renders the textbox part of a ComboBox control. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, string comboBoxText, Font font, Rectangle textBounds, TextFormatFlags flags, ComboBoxState state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, (int)state); } DrawBackground(g, bounds, state); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, comboBoxText, font, textBounds, textColor, flags); } ////// Renders the textbox part of a ComboBox control. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawDropDownButton(Graphics g, Rectangle bounds, ComboBoxState state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(ComboBoxElement.ClassName, ComboBoxElement.Part, (int)state); } else { visualStyleRenderer.SetParameters(ComboBoxElement.ClassName, ComboBoxElement.Part, (int)state); } visualStyleRenderer.DrawBackground(g, bounds); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Renders a ComboBox drop-down button. /// ///
Link Menu
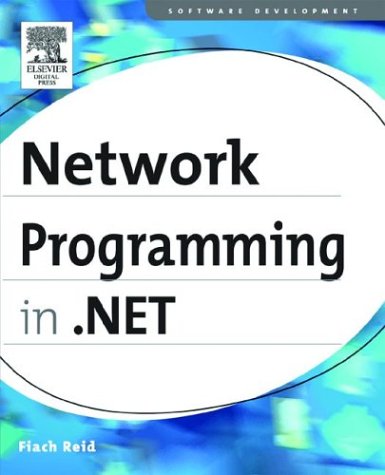
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DBSchemaRow.cs
- dbdatarecord.cs
- VerificationAttribute.cs
- PreviewKeyDownEventArgs.cs
- AsyncOperation.cs
- TreeViewDesigner.cs
- CacheDict.cs
- QilLiteral.cs
- RenderData.cs
- WindowsListViewGroupSubsetLink.cs
- TreeNodeStyleCollection.cs
- returneventsaver.cs
- TimelineGroup.cs
- OuterGlowBitmapEffect.cs
- RegexReplacement.cs
- DataGridColumn.cs
- SortDescription.cs
- ConditionalAttribute.cs
- MDIWindowDialog.cs
- StylusEventArgs.cs
- LinqDataSourceDisposeEventArgs.cs
- DbCommandTree.cs
- AssociationTypeEmitter.cs
- Image.cs
- HtmlInputText.cs
- GeneralTransform3DGroup.cs
- AssemblyNameProxy.cs
- ObservableCollection.cs
- MessageEventSubscriptionService.cs
- InternalBufferOverflowException.cs
- TextStore.cs
- DbSourceCommand.cs
- Pkcs7Signer.cs
- Pair.cs
- QilTargetType.cs
- AnimatedTypeHelpers.cs
- DefaultShape.cs
- ResourceDictionaryCollection.cs
- StructuredTypeEmitter.cs
- GlobalEventManager.cs
- CqlQuery.cs
- HScrollBar.cs
- EtwTrace.cs
- CustomTypeDescriptor.cs
- OptimizedTemplateContentHelper.cs
- FlowNode.cs
- LogEntry.cs
- lengthconverter.cs
- DateTimeFormatInfoScanner.cs
- ToolStripItem.cs
- MatrixAnimationUsingPath.cs
- XPathDocumentBuilder.cs
- DisplayInformation.cs
- AnnotationDocumentPaginator.cs
- CustomWebEventKey.cs
- SqlCacheDependencyDatabase.cs
- AttachedPropertyBrowsableAttribute.cs
- DataGridViewSelectedCellCollection.cs
- RolePrincipal.cs
- ServiceBusyException.cs
- CryptoHandle.cs
- SmtpMail.cs
- JapaneseLunisolarCalendar.cs
- ResourceWriter.cs
- TextProviderWrapper.cs
- WorkerRequest.cs
- DeploymentExceptionMapper.cs
- XmlSchemaSimpleContent.cs
- ValueTypeFixupInfo.cs
- SecurityRuntime.cs
- ImpersonateTokenRef.cs
- ObjectStorage.cs
- TransformerConfigurationWizardBase.cs
- ContractHandle.cs
- OrderedParallelQuery.cs
- RenamedEventArgs.cs
- StylusPointPropertyUnit.cs
- TextAnchor.cs
- SaveFileDialog.cs
- GenericIdentity.cs
- BrowserDefinition.cs
- DataServiceRequestException.cs
- XPathParser.cs
- TheQuery.cs
- Rules.cs
- TypeDependencyAttribute.cs
- Stroke.cs
- OutputCacheSettings.cs
- FtpCachePolicyElement.cs
- Regex.cs
- WebPartConnectionsConfigureVerb.cs
- DurationConverter.cs
- DirectoryInfo.cs
- AttributeUsageAttribute.cs
- GridToolTip.cs
- StrongNameSignatureInformation.cs
- HtmlButton.cs
- FontStretchConverter.cs
- MatrixStack.cs
- GlyphingCache.cs