Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / mediaclock.cs / 1 / mediaclock.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: mediaclock.cs // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.ComponentModel; using MS.Internal; using MS.Win32; using System.Windows.Media.Animation; using System.Windows.Media; using System.Windows.Media.Composition; using System.Windows.Markup; using System.Security.Permissions; using System.Security; using MS.Internal.PresentationCore; // SecurityHelper using System.Windows.Threading; using System.Runtime.InteropServices; using System.IO; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { #region MediaClock ////// Maintains run-time timing state for media (audio/video) objects. /// public class MediaClock : Clock { #region Constructors and Finalizers ////// Creates a MediaClock object. /// /// /// The MediaTimeline to use as a template. /// ////// The returned MediaClock doesn't have any children. /// protected internal MediaClock(MediaTimeline media) : base(media) {} #endregion #region Properties ////// Gets the MediaTimeline object that holds the description controlling the /// behavior of this clock. /// ////// The MediaTimeline object that holds the description controlling the /// behavior of this clock. /// public new MediaTimeline Timeline { get { return (MediaTimeline)base.Timeline; } } #endregion #region Clock Overrides ////// Returns True because Media has the potential to slip. /// ///True protected override bool GetCanSlip() { return true; } ////// Get the actual media time for slip synchronization /// protected override TimeSpan GetCurrentTimeCore() { if (_mediaPlayer != null) { return _mediaPlayer.Position; } else // Otherwise use base implementation { return base.GetCurrentTimeCore(); } } ////// Called when we are stopped. This is the same as pausing and seeking /// to the beginning. /// protected override void Stopped() { // Only perform the operation if we're controlling a player if (_mediaPlayer != null) { _mediaPlayer.SetSpeedRatio(0); _mediaPlayer.SetPosition(TimeSpan.FromTicks(0)); } } ////// Called when our speed changes. A discontinuous time movement may or /// may not have occurred. /// protected override void SpeedChanged() { [....](); } ////// Called when we have a discontinuous time movement, but no change in /// speed /// protected override void DiscontinuousTimeMovement() { [....](); } private void [....]() { // Only perform the operation if we're controlling a player if (_mediaPlayer != null) { double? currentSpeedProperty = this.CurrentGlobalSpeed; double currentSpeedValue = currentSpeedProperty.HasValue ? currentSpeedProperty.Value : 0; TimeSpan? currentTimeProperty = this.CurrentTime; TimeSpan currentTimeValue = currentTimeProperty.HasValue ? currentTimeProperty.Value : TimeSpan.Zero; // If speed was potentially changed to 0, make sure we set media's speed to 0 (e.g. pause) before // setting the position to the target frame. Otherwise, the media's scrubbing mechanism would // not work correctly, because scrubbing requires media to be paused by the time it is seeked. if (currentSpeedValue == 0) { _mediaPlayer.SetSpeedRatio(currentSpeedValue); _mediaPlayer.SetPosition(currentTimeValue); } else { // In the case where speed != 0, we first want to set the position and then the speed. // This is because if we were previously paused, we want to be at the right position // before we begin to play. _mediaPlayer.SetPosition(currentTimeValue); _mediaPlayer.SetSpeedRatio(currentSpeedValue); } } } ////// Returns true if this timeline needs continuous frames. /// This is a hint that we should keep updating our time during the active period. /// ///internal override bool NeedsTicksWhenActive { get { return true; } } /// /// The instance of media that this clock is driving /// internal MediaPlayer Player { get { return _mediaPlayer; } set { MediaPlayer oldPlayer = _mediaPlayer; MediaPlayer newPlayer = value; // avoid inifite loops if (newPlayer != oldPlayer) { _mediaPlayer = newPlayer; // Disassociate the old player if (oldPlayer != null) { oldPlayer.Clock = null; } // Associate the new player if (newPlayer != null) { newPlayer.Clock = this; Uri baseUri = ((IUriContext)Timeline).BaseUri; Uri toPlay = null; // ignore pack URIs for now (see work items 45396 and 41636) if (baseUri != null && baseUri.Scheme != System.IO.Packaging.PackUriHelper.UriSchemePack && !Timeline.Source.IsAbsoluteUri) { toPlay = new Uri(baseUri, Timeline.Source); } else { // // defaults to app domain base if Timeline.Source is // relative // toPlay = Timeline.Source; } // we need to [....] to the current state of the clock newPlayer.SetSource(toPlay); SpeedChanged(); } } } } #endregion #region Private Data members ////// MediaPlayer -- holds all the precious resource references /// private MediaPlayer _mediaPlayer; #endregion } #endregion }; // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: mediaclock.cs // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.ComponentModel; using MS.Internal; using MS.Win32; using System.Windows.Media.Animation; using System.Windows.Media; using System.Windows.Media.Composition; using System.Windows.Markup; using System.Security.Permissions; using System.Security; using MS.Internal.PresentationCore; // SecurityHelper using System.Windows.Threading; using System.Runtime.InteropServices; using System.IO; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { #region MediaClock ////// Maintains run-time timing state for media (audio/video) objects. /// public class MediaClock : Clock { #region Constructors and Finalizers ////// Creates a MediaClock object. /// /// /// The MediaTimeline to use as a template. /// ////// The returned MediaClock doesn't have any children. /// protected internal MediaClock(MediaTimeline media) : base(media) {} #endregion #region Properties ////// Gets the MediaTimeline object that holds the description controlling the /// behavior of this clock. /// ////// The MediaTimeline object that holds the description controlling the /// behavior of this clock. /// public new MediaTimeline Timeline { get { return (MediaTimeline)base.Timeline; } } #endregion #region Clock Overrides ////// Returns True because Media has the potential to slip. /// ///True protected override bool GetCanSlip() { return true; } ////// Get the actual media time for slip synchronization /// protected override TimeSpan GetCurrentTimeCore() { if (_mediaPlayer != null) { return _mediaPlayer.Position; } else // Otherwise use base implementation { return base.GetCurrentTimeCore(); } } ////// Called when we are stopped. This is the same as pausing and seeking /// to the beginning. /// protected override void Stopped() { // Only perform the operation if we're controlling a player if (_mediaPlayer != null) { _mediaPlayer.SetSpeedRatio(0); _mediaPlayer.SetPosition(TimeSpan.FromTicks(0)); } } ////// Called when our speed changes. A discontinuous time movement may or /// may not have occurred. /// protected override void SpeedChanged() { [....](); } ////// Called when we have a discontinuous time movement, but no change in /// speed /// protected override void DiscontinuousTimeMovement() { [....](); } private void [....]() { // Only perform the operation if we're controlling a player if (_mediaPlayer != null) { double? currentSpeedProperty = this.CurrentGlobalSpeed; double currentSpeedValue = currentSpeedProperty.HasValue ? currentSpeedProperty.Value : 0; TimeSpan? currentTimeProperty = this.CurrentTime; TimeSpan currentTimeValue = currentTimeProperty.HasValue ? currentTimeProperty.Value : TimeSpan.Zero; // If speed was potentially changed to 0, make sure we set media's speed to 0 (e.g. pause) before // setting the position to the target frame. Otherwise, the media's scrubbing mechanism would // not work correctly, because scrubbing requires media to be paused by the time it is seeked. if (currentSpeedValue == 0) { _mediaPlayer.SetSpeedRatio(currentSpeedValue); _mediaPlayer.SetPosition(currentTimeValue); } else { // In the case where speed != 0, we first want to set the position and then the speed. // This is because if we were previously paused, we want to be at the right position // before we begin to play. _mediaPlayer.SetPosition(currentTimeValue); _mediaPlayer.SetSpeedRatio(currentSpeedValue); } } } ////// Returns true if this timeline needs continuous frames. /// This is a hint that we should keep updating our time during the active period. /// ///internal override bool NeedsTicksWhenActive { get { return true; } } /// /// The instance of media that this clock is driving /// internal MediaPlayer Player { get { return _mediaPlayer; } set { MediaPlayer oldPlayer = _mediaPlayer; MediaPlayer newPlayer = value; // avoid inifite loops if (newPlayer != oldPlayer) { _mediaPlayer = newPlayer; // Disassociate the old player if (oldPlayer != null) { oldPlayer.Clock = null; } // Associate the new player if (newPlayer != null) { newPlayer.Clock = this; Uri baseUri = ((IUriContext)Timeline).BaseUri; Uri toPlay = null; // ignore pack URIs for now (see work items 45396 and 41636) if (baseUri != null && baseUri.Scheme != System.IO.Packaging.PackUriHelper.UriSchemePack && !Timeline.Source.IsAbsoluteUri) { toPlay = new Uri(baseUri, Timeline.Source); } else { // // defaults to app domain base if Timeline.Source is // relative // toPlay = Timeline.Source; } // we need to [....] to the current state of the clock newPlayer.SetSource(toPlay); SpeedChanged(); } } } } #endregion #region Private Data members ////// MediaPlayer -- holds all the precious resource references /// private MediaPlayer _mediaPlayer; #endregion } #endregion }; // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
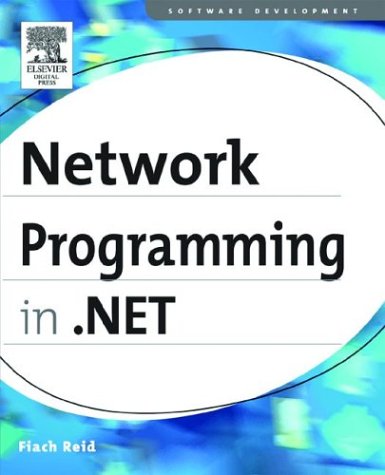
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DebugHandleTracker.cs
- TypefaceCollection.cs
- DataSourceBooleanViewSchemaConverter.cs
- XmlTextReader.cs
- ParamArrayAttribute.cs
- IntegerFacetDescriptionElement.cs
- XmlSchemaValidationException.cs
- XamlFilter.cs
- DataGridPagerStyle.cs
- ConnectionManagementElementCollection.cs
- BulletedList.cs
- DeclarativeCatalogPart.cs
- ApplicationCommands.cs
- ConfigXmlReader.cs
- ModifierKeysConverter.cs
- MatrixAnimationUsingPath.cs
- TypeUtils.cs
- StructuredType.cs
- ObservableCollectionDefaultValueFactory.cs
- FixedSOMElement.cs
- DoubleLinkListEnumerator.cs
- ColumnMapVisitor.cs
- httpapplicationstate.cs
- InputElement.cs
- BinaryParser.cs
- TypeListConverter.cs
- CheckBox.cs
- SelectionHighlightInfo.cs
- CharAnimationUsingKeyFrames.cs
- TextureBrush.cs
- DataObjectCopyingEventArgs.cs
- RecognizedPhrase.cs
- errorpatternmatcher.cs
- VectorCollection.cs
- FramingDecoders.cs
- BaseTransportHeaders.cs
- CodeTryCatchFinallyStatement.cs
- LoginCancelEventArgs.cs
- RadioButtonStandardAdapter.cs
- IISUnsafeMethods.cs
- XmlReaderSettings.cs
- PerfProviderCollection.cs
- LeaseManager.cs
- cookie.cs
- GeneralTransformGroup.cs
- RegexWriter.cs
- FirstMatchCodeGroup.cs
- RichTextBoxAutomationPeer.cs
- MenuItem.cs
- TagElement.cs
- Preprocessor.cs
- x509store.cs
- PolyBezierSegment.cs
- DescendentsWalker.cs
- PersonalizableTypeEntry.cs
- Rect3D.cs
- ObjectFullSpanRewriter.cs
- Error.cs
- VariableExpressionConverter.cs
- Shape.cs
- ReceiveActivityValidator.cs
- EventMemberCodeDomSerializer.cs
- PageRequestManager.cs
- DeferredRunTextReference.cs
- URLMembershipCondition.cs
- LocationInfo.cs
- SingleAnimationBase.cs
- printdlgexmarshaler.cs
- PolicyLevel.cs
- PtsCache.cs
- TreeIterator.cs
- ReferencedType.cs
- DataGridViewLinkCell.cs
- ToolStripItemDataObject.cs
- CodeDefaultValueExpression.cs
- BufferedStream2.cs
- ContractReference.cs
- SettingsPropertyValue.cs
- FormViewPageEventArgs.cs
- ContentPlaceHolder.cs
- TextRangeEdit.cs
- InternalEnumValidator.cs
- DefinitionUpdate.cs
- KeySplineConverter.cs
- EntityCommandCompilationException.cs
- ActivityBindForm.Designer.cs
- EntityKeyElement.cs
- CheckBoxAutomationPeer.cs
- XMLUtil.cs
- Utils.cs
- Compiler.cs
- AnnotationHelper.cs
- SqlBulkCopy.cs
- WmlLiteralTextAdapter.cs
- IconBitmapDecoder.cs
- WrapPanel.cs
- ZoneMembershipCondition.cs
- EndpointFilterProvider.cs
- CheckedPointers.cs
- DetailsViewUpdateEventArgs.cs