Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewHitTestInfo.cs / 1 / DataGridViewHitTestInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Globalization; namespace System.Windows.Forms { public partial class DataGridView { ///public sealed class HitTestInfo { internal DataGridViewHitTestType type = DataGridViewHitTestType.None; //internal DataGridViewHitTestTypeCloseEdge edge = DataGridViewHitTestTypeCloseEdge.None; internal DataGridViewHitTestTypeInternal typeInternal = DataGridViewHitTestTypeInternal.None; internal int row; internal int col; internal int adjacentRow; internal int adjacentCol; internal int mouseBarOffset; internal int rowStart; internal int colStart; /// /// /// public static readonly HitTestInfo Nowhere = new HitTestInfo(); internal HitTestInfo() { this.type = DataGridViewHitTestType.None; this.typeInternal = DataGridViewHitTestTypeInternal.None; //this.edge = DataGridViewHitTestTypeCloseEdge.None; this.row = this.col = -1; this.rowStart = this.colStart = -1; this.adjacentRow = this.adjacentCol = -1; } ///Allows the ///object to inform you the /// extent of the grid. /// /// public int ColumnIndex { get { return this.col; } } ///Gets the number of the clicked column. ////// /// public int RowIndex { get { return this.row; } } ///Gets the /// number of the clicked row. ////// /// public int ColumnX { get { return this.colStart; } } ///Gets the left edge of the column. ////// /// public int RowY { get { return this.rowStart; } } ///Gets the top edge of the row. ////// /// public DataGridViewHitTestType Type { get { return this.type; } } ///Gets the part of the ///control, other than the row or column, that was /// clicked. /// /// public override bool Equals(object value) { HitTestInfo hti = value as HitTestInfo; if (hti != null) { return (this.type == hti.type && this.row == hti.row && this.col == hti.col); } return false; } ///Indicates whether two objects are identical. ////// /// public override int GetHashCode() { return WindowsFormsUtils.GetCombinedHashCodes((int) this.type, this.row, this.col); } ///Gets the hash code for the ///instance. /// /// public override string ToString() { return "{ Type:" + type.ToString() + ", Column:" + col.ToString(CultureInfo.CurrentCulture) + ", Row:" + row.ToString(CultureInfo.CurrentCulture) + " }"; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Gets the type, column number and row number. ///
Link Menu
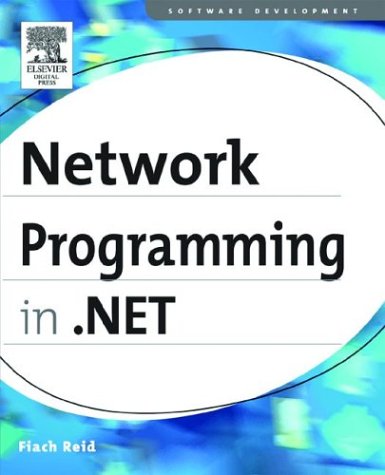
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BitmapEffectDrawing.cs
- DbReferenceCollection.cs
- LowerCaseStringConverter.cs
- DetailsViewDeletedEventArgs.cs
- CollectionViewGroup.cs
- ListenerConfig.cs
- State.cs
- AppDomainUnloadedException.cs
- ResXFileRef.cs
- FeatureSupport.cs
- SelectionRangeConverter.cs
- InfoCardCryptoHelper.cs
- RuleInfoComparer.cs
- Vector.cs
- NavigationHelper.cs
- Light.cs
- WrappedOptions.cs
- AutomationIdentifierGuids.cs
- SqlProvider.cs
- ResourceWriter.cs
- ExtendedPropertyDescriptor.cs
- CompilationUnit.cs
- FixUpCollection.cs
- DebuggerAttributes.cs
- WmiInstallComponent.cs
- UrlPropertyAttribute.cs
- SqlMethodAttribute.cs
- GenericPrincipal.cs
- ContentHostHelper.cs
- brushes.cs
- NavigationService.cs
- EntryPointNotFoundException.cs
- TypeToken.cs
- DnsPermission.cs
- ProviderConnectionPoint.cs
- JpegBitmapDecoder.cs
- FontNamesConverter.cs
- TextBox.cs
- NameValuePermission.cs
- EmptyControlCollection.cs
- SqlWriter.cs
- UnsafeNativeMethodsPenimc.cs
- XmlNamespaceMappingCollection.cs
- HttpContextBase.cs
- SignedPkcs7.cs
- DataMemberConverter.cs
- AuthenticationModulesSection.cs
- Style.cs
- BitmapEffectRenderDataResource.cs
- WebZone.cs
- WebControlsSection.cs
- XmlWriter.cs
- BindingContext.cs
- StretchValidation.cs
- HashCryptoHandle.cs
- CryptoStream.cs
- CodeMemberField.cs
- SqlNodeTypeOperators.cs
- DesignBindingValueUIHandler.cs
- TextComposition.cs
- X509UI.cs
- HtmlInputText.cs
- ClientSettingsProvider.cs
- ToolBar.cs
- XmlDataContract.cs
- TextEditorCharacters.cs
- InternalTypeHelper.cs
- BitmapEffectRenderDataResource.cs
- TextShapeableCharacters.cs
- __Error.cs
- MatrixConverter.cs
- TargetControlTypeCache.cs
- BehaviorDragDropEventArgs.cs
- CompositeFontParser.cs
- Transform.cs
- SelectionGlyph.cs
- DispatcherOperation.cs
- StyleXamlParser.cs
- XmlAtomicValue.cs
- IconConverter.cs
- ApplicationHost.cs
- Crc32Helper.cs
- SimpleWorkerRequest.cs
- ImmComposition.cs
- CultureTableRecord.cs
- SystemInfo.cs
- TimersDescriptionAttribute.cs
- EventProvider.cs
- WinEventWrap.cs
- WebPartsPersonalization.cs
- _SingleItemRequestCache.cs
- HtmlControlAdapter.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- X509Utils.cs
- XmlNamespaceMappingCollection.cs
- EventTrigger.cs
- ActivityFunc.cs
- HashMembershipCondition.cs
- AtomMaterializer.cs
- DefaultBinder.cs