Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlInputText.cs / 3 / HtmlInputText.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputText.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System.ComponentModel; using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ DefaultEvent("ServerChange"), SupportsEventValidation, ValidationProperty("Value"), ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlInputText : HtmlInputControl, IPostBackDataHandler { private static readonly object EventServerChange = new object(); /* * Creates an intrinsic Html INPUT type=text control. */ public HtmlInputText() : base("text") { } /* * Creates an intrinsic Html INPUT type=text control. */ ////// The ////// class defines the methods, properties, and events for the HtmlInputText server /// control. This class allows programmatic access to the HTML <input type= /// text> /// and <input type= /// password> elements on the server. /// /// public HtmlInputText(string type) : base(type) { } /* * The property for the maximum characters allowed. */ ////// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int MaxLength { get { string s = (string)ViewState["maxlength"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["maxlength"] = MapIntegerAttributeToString(value); } } // /* * The property for the width of the TextBox in characters. */ ////// Gets or sets the maximum number of characters that /// can be typed into the text box. /// ////// [ WebCategory("Appearance"), DefaultValue(-1), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Size { get { string s = Attributes["size"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["size"] = MapIntegerAttributeToString(value); } } /* * Value property. */ ////// Gets or sets the width of a text box, in characters. /// ////// public override string Value { get { string s = Attributes["value"]; return((s != null) ? s : String.Empty); } set { Attributes["value"] = MapStringAttributeToString(value); } } [ WebCategory("Action"), WebSysDescription(SR.HtmlInputText_ServerChange) ] public event EventHandler ServerChange { add { Events.AddHandler(EventServerChange, value); } remove { Events.RemoveHandler(EventServerChange, value); } } /* * Method used to raise the OnServerChange event. */ ////// Gets or sets the /// contents of a text box. /// ////// protected virtual void OnServerChange(EventArgs e) { EventHandler handler = (EventHandler)Events[EventServerChange]; if (handler != null) handler(this, e); } /* * */ ////// /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); bool disabled = Disabled; if (!disabled && Page != null) { Page.RegisterEnabledControl(this); } // if no change handler, no need to save posted property unless we are disabled; // if ((!disabled && Events[EventServerChange] == null) || Type.Equals("password", StringComparison.OrdinalIgnoreCase)) { ViewState.SetItemDirty("value", false); } } protected override void RenderAttributes(HtmlTextWriter writer) { base.RenderAttributes(writer); if (Page != null) { Page.ClientScript.RegisterForEventValidation(RenderedNameAttribute); } } /* * Method of IPostBackDataHandler interface to process posted data. * InputText process a newly posted value. */ ////// /// bool IPostBackDataHandler.LoadPostData(string postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } ////// /// protected virtual bool LoadPostData(string postDataKey, NameValueCollection postCollection) { string current = Value; string inputString = postCollection.GetValues(postDataKey)[0]; if (!current.Equals(inputString)) { ValidateEvent(postDataKey); Value = inputString; return true; } return false; } /* * Method of IPostBackDataHandler interface which is invoked whenever posted data * for a control has changed. InputText fires an OnServerChange event. */ ////// /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ////// /// protected virtual void RaisePostDataChangedEvent() { OnServerChange(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
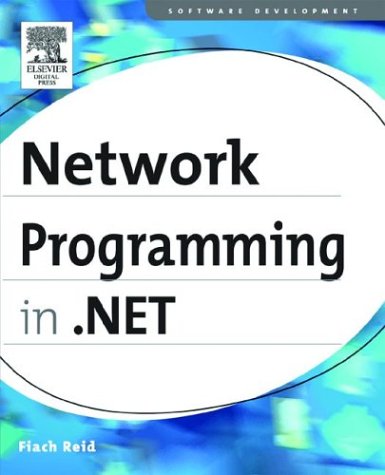
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DependencyPropertyConverter.cs
- ExpressionEditorAttribute.cs
- RedistVersionInfo.cs
- MimeFormReflector.cs
- CmsUtils.cs
- ViewStateModeByIdAttribute.cs
- TabControl.cs
- Pts.cs
- ColorAnimationUsingKeyFrames.cs
- StaticResourceExtension.cs
- VirtualPathProvider.cs
- PhoneCallDesigner.cs
- AutomationPattern.cs
- BamlTreeNode.cs
- DateTimeConstantAttribute.cs
- RenameRuleObjectDialog.Designer.cs
- FormViewUpdatedEventArgs.cs
- ObjectDataSourceMethodEditor.cs
- DoWorkEventArgs.cs
- XmlQueryContext.cs
- DbProviderFactoriesConfigurationHandler.cs
- VectorValueSerializer.cs
- TypeTypeConverter.cs
- ReflectTypeDescriptionProvider.cs
- WpfPayload.cs
- TextParagraph.cs
- SiteMapPath.cs
- XmlSchemaComplexContent.cs
- RequestContext.cs
- _IPv6Address.cs
- WasEndpointConfigContainer.cs
- wgx_exports.cs
- XmlLinkedNode.cs
- ApplyHostConfigurationBehavior.cs
- JsonReader.cs
- XmlDataSource.cs
- SQLBinary.cs
- SafeRightsManagementEnvironmentHandle.cs
- BlurBitmapEffect.cs
- XMLDiffLoader.cs
- CopyOnWriteList.cs
- NavigationEventArgs.cs
- GradientBrush.cs
- LinqTreeNodeEvaluator.cs
- GiveFeedbackEventArgs.cs
- DataSourceUtil.cs
- hebrewshape.cs
- SafeEventLogReadHandle.cs
- ComPlusTraceRecord.cs
- ScrollBar.cs
- DataGridViewCellStateChangedEventArgs.cs
- ToolStripItemTextRenderEventArgs.cs
- ClassDataContract.cs
- Encoder.cs
- ZipIOLocalFileDataDescriptor.cs
- MonthChangedEventArgs.cs
- JsonDeserializer.cs
- XamlInt32CollectionSerializer.cs
- DataBindingList.cs
- DecimalAnimation.cs
- Style.cs
- SHA256Managed.cs
- TreeNode.cs
- HandleRef.cs
- MSG.cs
- Boolean.cs
- XmlWriter.cs
- ReadWriteControlDesigner.cs
- securitycriticaldata.cs
- DataSourceCacheDurationConverter.cs
- ZipFileInfoCollection.cs
- ValueConversionAttribute.cs
- VScrollProperties.cs
- InkCanvasSelectionAdorner.cs
- XmlChildNodes.cs
- InfiniteIntConverter.cs
- IntellisenseTextBox.cs
- MSG.cs
- TextTreeTextBlock.cs
- GlobalizationAssembly.cs
- SqlInternalConnectionTds.cs
- DbConnectionStringBuilder.cs
- _Rfc2616CacheValidators.cs
- CompositeControlDesigner.cs
- Crypto.cs
- DataGridBoolColumn.cs
- DatePickerTextBox.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- BaseComponentEditor.cs
- TableLayoutStyleCollection.cs
- AvtEvent.cs
- SchemaElementLookUpTableEnumerator.cs
- TypeDelegator.cs
- SignedPkcs7.cs
- NetworkAddressChange.cs
- QilBinary.cs
- SecurityTokenSerializer.cs
- SafeNativeMethodsMilCoreApi.cs
- MediaContextNotificationWindow.cs
- TranslateTransform3D.cs