Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Xml / System / Xml / Core / SecureStringHasher.cs / 1 / SecureStringHasher.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Xml { // SecureStringHasher is a hash code provider for strings. The hash codes calculation starts with a seed (hasCodeRandomizer) which is usually // different for each instance of SecureStringHasher. Since the hash code depend on the seed, the chance of hashtable DoS attack in case when // someone passes in lots of strings that hash to the same hash code is greatly reduced. // The SecureStringHasher implements IEqualityComparer for strings and therefore can be used in generic IDictionary. internal class SecureStringHasher : IEqualityComparer{ int hashCodeRandomizer; public SecureStringHasher() { this.hashCodeRandomizer = Environment.TickCount; } public SecureStringHasher( int hashCodeRandomizer ) { this.hashCodeRandomizer = hashCodeRandomizer; } public int Compare( String x, String y ) { return String.Compare(x, y, StringComparison.Ordinal); } public bool Equals( String x, String y ) { return String.Equals( x, y, StringComparison.Ordinal ); } public int GetHashCode( String key ) { int hashCode = hashCodeRandomizer; // use key.Length to eliminate the rangecheck for ( int i = 0; i < key.Length; i++ ) { hashCode += ( hashCode << 7 ) ^ key[i]; } // mix it a bit more hashCode -= hashCode >> 17; hashCode -= hashCode >> 11; hashCode -= hashCode >> 5; return hashCode; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Xml { // SecureStringHasher is a hash code provider for strings. The hash codes calculation starts with a seed (hasCodeRandomizer) which is usually // different for each instance of SecureStringHasher. Since the hash code depend on the seed, the chance of hashtable DoS attack in case when // someone passes in lots of strings that hash to the same hash code is greatly reduced. // The SecureStringHasher implements IEqualityComparer for strings and therefore can be used in generic IDictionary. internal class SecureStringHasher : IEqualityComparer{ int hashCodeRandomizer; public SecureStringHasher() { this.hashCodeRandomizer = Environment.TickCount; } public SecureStringHasher( int hashCodeRandomizer ) { this.hashCodeRandomizer = hashCodeRandomizer; } public int Compare( String x, String y ) { return String.Compare(x, y, StringComparison.Ordinal); } public bool Equals( String x, String y ) { return String.Equals( x, y, StringComparison.Ordinal ); } public int GetHashCode( String key ) { int hashCode = hashCodeRandomizer; // use key.Length to eliminate the rangecheck for ( int i = 0; i < key.Length; i++ ) { hashCode += ( hashCode << 7 ) ^ key[i]; } // mix it a bit more hashCode -= hashCode >> 17; hashCode -= hashCode >> 11; hashCode -= hashCode >> 5; return hashCode; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
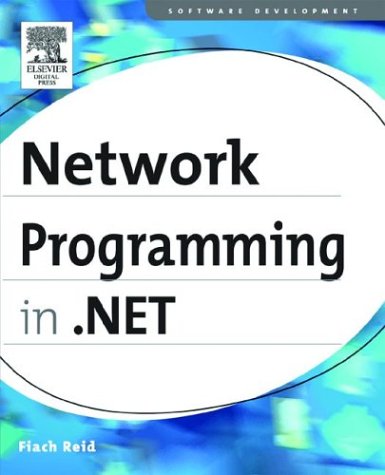
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrintPreviewDialog.cs
- CustomWebEventKey.cs
- IgnoreDataMemberAttribute.cs
- InheritanceAttribute.cs
- WmlTextViewAdapter.cs
- CellConstantDomain.cs
- TemplateModeChangedEventArgs.cs
- AuthenticationModulesSection.cs
- BinaryWriter.cs
- TemplateBindingExpressionConverter.cs
- RotationValidation.cs
- VariantWrapper.cs
- ProtocolViolationException.cs
- ConnectionInterfaceCollection.cs
- MailWebEventProvider.cs
- ToolStripControlHost.cs
- RC2.cs
- DragCompletedEventArgs.cs
- RankException.cs
- InfoCardSymmetricAlgorithm.cs
- XmlnsCompatibleWithAttribute.cs
- FixedLineResult.cs
- KnownTypeDataContractResolver.cs
- UnrecognizedAssertionsBindingElement.cs
- CodeEventReferenceExpression.cs
- GifBitmapDecoder.cs
- CodeMemberEvent.cs
- RoutedEvent.cs
- RpcCryptoContext.cs
- Compiler.cs
- CompilerCollection.cs
- NavigationEventArgs.cs
- MULTI_QI.cs
- TextContainerChangeEventArgs.cs
- Cursor.cs
- _NetworkingPerfCounters.cs
- FaultPropagationRecord.cs
- DiscardableAttribute.cs
- PrinterResolution.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- State.cs
- QilCloneVisitor.cs
- WebPartTransformerAttribute.cs
- XamlToRtfWriter.cs
- ArgumentOutOfRangeException.cs
- SoapSchemaImporter.cs
- CommonObjectSecurity.cs
- Parameter.cs
- TPLETWProvider.cs
- HtmlInputText.cs
- KeyValuePairs.cs
- WinFormsUtils.cs
- AutomationPeer.cs
- TextServicesLoader.cs
- XmlSchemaSimpleTypeRestriction.cs
- CodeTypeReferenceSerializer.cs
- CodeMethodReturnStatement.cs
- TypedLocationWrapper.cs
- DisplayNameAttribute.cs
- ResourceLoader.cs
- ApplicationInterop.cs
- RandomNumberGenerator.cs
- UpdateTracker.cs
- PersonalizableAttribute.cs
- Menu.cs
- BinaryUtilClasses.cs
- EUCJPEncoding.cs
- GridEntryCollection.cs
- HttpWriter.cs
- ObjectStateFormatter.cs
- ResourceManager.cs
- SecurityTokenAuthenticator.cs
- RemotingConfiguration.cs
- ParserExtension.cs
- DataException.cs
- ValueTable.cs
- SafeCryptoHandles.cs
- EmbeddedObject.cs
- RefExpr.cs
- HttpRequestWrapper.cs
- ToolStripArrowRenderEventArgs.cs
- PriorityBinding.cs
- BezierSegment.cs
- ColorContextHelper.cs
- IsolatedStorageException.cs
- XmlDictionaryReaderQuotas.cs
- ButtonBase.cs
- _OverlappedAsyncResult.cs
- ObjectViewListener.cs
- XmlSchemaSearchPattern.cs
- BamlWriter.cs
- WebScriptMetadataInstanceContextProvider.cs
- ObjectDisposedException.cs
- WebPartDisplayModeEventArgs.cs
- DataListAutoFormat.cs
- ExpressionBuilderCollection.cs
- OneOfScalarConst.cs
- assemblycache.cs
- TabPanel.cs
- WebReferencesBuildProvider.cs