Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / LongSumAggregationOperator.cs / 1305376 / LongSumAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // LongSumAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for longs. /// internal sealed class LongSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal LongSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override long InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. long sum = 0L; while (enumerator.MoveNext()) { checked { sum += enumerator.Current; } } return sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new LongSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class LongSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal LongSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref long currentElement) { long element = default(long); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. long tempSum = 0L; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); checked { tempSum += element; } } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = tempSum; return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // LongSumAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for longs. /// internal sealed class LongSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal LongSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override long InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. long sum = 0L; while (enumerator.MoveNext()) { checked { sum += enumerator.Current; } } return sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new LongSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class LongSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal LongSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref long currentElement) { long element = default(long); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. long tempSum = 0L; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); checked { tempSum += element; } } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = tempSum; return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
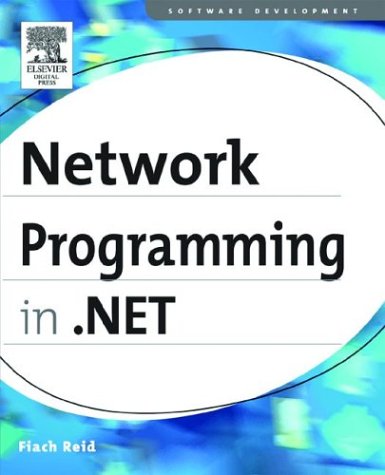
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EditorAttribute.cs
- ChannelDemuxer.cs
- MemberListBinding.cs
- DataGridViewTextBoxColumn.cs
- EntityDataSourceSelectingEventArgs.cs
- HashAlgorithm.cs
- ContainerParagraph.cs
- HeaderCollection.cs
- UnsafeNativeMethods.cs
- CodeChecksumPragma.cs
- ModulesEntry.cs
- StrongNameIdentityPermission.cs
- XmlKeywords.cs
- HttpClientCertificate.cs
- SmiMetaDataProperty.cs
- AssociatedControlConverter.cs
- NodeFunctions.cs
- ToolStripPanelCell.cs
- RectangleHotSpot.cs
- XPathParser.cs
- SupportsEventValidationAttribute.cs
- ParallelQuery.cs
- DeflateEmulationStream.cs
- Graphics.cs
- EventLog.cs
- TrustLevelCollection.cs
- BookmarkCallbackWrapper.cs
- X509Chain.cs
- InputBuffer.cs
- DictionaryTraceRecord.cs
- Merger.cs
- WebHeaderCollection.cs
- StretchValidation.cs
- LinearGradientBrush.cs
- LeaseManager.cs
- EmbeddedMailObject.cs
- ThreadExceptionEvent.cs
- GenerateHelper.cs
- CacheDependency.cs
- CompilerErrorCollection.cs
- VariableExpressionConverter.cs
- BamlStream.cs
- DockPanel.cs
- columnmapfactory.cs
- UserMapPath.cs
- RoleBoolean.cs
- Geometry3D.cs
- RegionInfo.cs
- TextParaLineResult.cs
- DataBindingHandlerAttribute.cs
- TextDocumentView.cs
- InputLangChangeRequestEvent.cs
- HMACSHA384.cs
- IconHelper.cs
- FixedNode.cs
- BaseDataBoundControl.cs
- StaticTextPointer.cs
- SymDocumentType.cs
- TableStyle.cs
- cache.cs
- DesignObjectWrapper.cs
- MethodRental.cs
- DockPattern.cs
- InputLanguageSource.cs
- Viewport2DVisual3D.cs
- TextureBrush.cs
- _NtlmClient.cs
- RuntimeResourceSet.cs
- DelegatedStream.cs
- Package.cs
- IriParsingElement.cs
- ParseChildrenAsPropertiesAttribute.cs
- ChildTable.cs
- TemplateKey.cs
- Menu.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- MultipartIdentifier.cs
- DefaultAsyncDataDispatcher.cs
- DataServiceRequest.cs
- WindowsGraphics.cs
- DurationConverter.cs
- ValidationRuleCollection.cs
- EnterpriseServicesHelper.cs
- XmlReaderSettings.cs
- AttachedAnnotationChangedEventArgs.cs
- ToolStripOverflowButton.cs
- HttpHeaderCollection.cs
- FixedTextView.cs
- BitmapEncoder.cs
- SignerInfo.cs
- SystemColors.cs
- CultureInfo.cs
- CodeExpressionStatement.cs
- UnionCqlBlock.cs
- VariableExpressionConverter.cs
- ReadWriteSpinLock.cs
- DataGridRow.cs
- DataSourceCacheDurationConverter.cs
- ElementNotAvailableException.cs
- ChangePasswordAutoFormat.cs