Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Annotations / AnnotationResourceChangedEventArgs.cs / 1305600 / AnnotationResourceChangedEventArgs.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // AnnotationResourceChangedEvents are fired by an Annotation when a // AnnotationResource it contains has been added, removed or modified in // some way. The event includes the annotation, the AnnotationResource, // and what action was taken on the resoure. // // Spec: http://team/sites/ag/Specifications/Simplifying%20Store%20Cache%20Model.doc // // History: // 06/30/2004: rruiz: Added new event (args and handler) for the new // Object Model design. //----------------------------------------------------------------------------- using System; using System.ComponentModel; namespace System.Windows.Annotations { ////// Delegate for handlers of the AnnotationResourceChanged event on Annotation. /// /// the annotation firing the event /// args describing the Resource and the action taken public delegate void AnnotationResourceChangedEventHandler(Object sender, AnnotationResourceChangedEventArgs e); ////// Event args for changes to an Annotation's Resources. This class includes /// the annotation that fired the event, the Resource that was changed, and /// what action was taken on the Resource - added, removed or modified. /// public sealed class AnnotationResourceChangedEventArgs : EventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of AnnotationResourceChangedEventArgs. /// /// the Annotation firing the event /// the action taken on the Resource /// the Resource that was changed ///annotation or action is null ///action is not a valid value from AnnotationAction public AnnotationResourceChangedEventArgs(Annotation annotation, AnnotationAction action, AnnotationResource resource) { // The resource parameter can be null here - it is possible to add a null to // the list of resources and we must fire an event signalling a change in the collection. if (annotation == null) { throw new ArgumentNullException("annotation"); } if (action < AnnotationAction.Added || action > AnnotationAction.Modified) { throw new InvalidEnumArgumentException("action", (int)action, typeof(AnnotationAction)); } _annotation = annotation; _resource = resource; _action = action; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// The Annotation that fired the event. /// public Annotation Annotation { get { return _annotation; } } ////// The Resource that was changed. /// public AnnotationResource Resource { get { return _resource; } } ////// The action that was taken on the Resource. /// public AnnotationAction Action { get { return _action; } } #endregion Public Properties //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields ////// The annotation that fired the event. /// private Annotation _annotation; ////// The Resource that was changed. /// private AnnotationResource _resource; ////// The action taken on the Resource /// private AnnotationAction _action; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // AnnotationResourceChangedEvents are fired by an Annotation when a // AnnotationResource it contains has been added, removed or modified in // some way. The event includes the annotation, the AnnotationResource, // and what action was taken on the resoure. // // Spec: http://team/sites/ag/Specifications/Simplifying%20Store%20Cache%20Model.doc // // History: // 06/30/2004: rruiz: Added new event (args and handler) for the new // Object Model design. //----------------------------------------------------------------------------- using System; using System.ComponentModel; namespace System.Windows.Annotations { ////// Delegate for handlers of the AnnotationResourceChanged event on Annotation. /// /// the annotation firing the event /// args describing the Resource and the action taken public delegate void AnnotationResourceChangedEventHandler(Object sender, AnnotationResourceChangedEventArgs e); ////// Event args for changes to an Annotation's Resources. This class includes /// the annotation that fired the event, the Resource that was changed, and /// what action was taken on the Resource - added, removed or modified. /// public sealed class AnnotationResourceChangedEventArgs : EventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of AnnotationResourceChangedEventArgs. /// /// the Annotation firing the event /// the action taken on the Resource /// the Resource that was changed ///annotation or action is null ///action is not a valid value from AnnotationAction public AnnotationResourceChangedEventArgs(Annotation annotation, AnnotationAction action, AnnotationResource resource) { // The resource parameter can be null here - it is possible to add a null to // the list of resources and we must fire an event signalling a change in the collection. if (annotation == null) { throw new ArgumentNullException("annotation"); } if (action < AnnotationAction.Added || action > AnnotationAction.Modified) { throw new InvalidEnumArgumentException("action", (int)action, typeof(AnnotationAction)); } _annotation = annotation; _resource = resource; _action = action; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// The Annotation that fired the event. /// public Annotation Annotation { get { return _annotation; } } ////// The Resource that was changed. /// public AnnotationResource Resource { get { return _resource; } } ////// The action that was taken on the Resource. /// public AnnotationAction Action { get { return _action; } } #endregion Public Properties //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields ////// The annotation that fired the event. /// private Annotation _annotation; ////// The Resource that was changed. /// private AnnotationResource _resource; ////// The action taken on the Resource /// private AnnotationAction _action; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
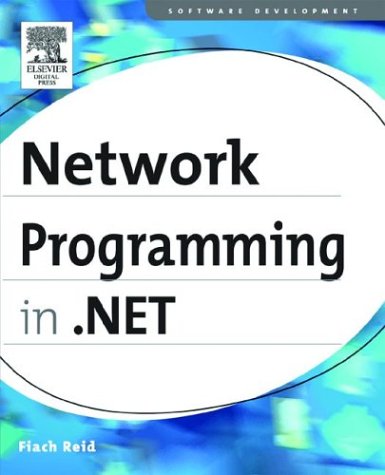
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewCellMouseEventArgs.cs
- EndpointConfigContainer.cs
- DefaultValueAttribute.cs
- ByteStream.cs
- LayoutEditorPart.cs
- DateTimeConverter.cs
- OracleBinary.cs
- DataBindingList.cs
- WCFBuildProvider.cs
- ProfilePropertySettings.cs
- BamlLocalizableResource.cs
- Decorator.cs
- Bits.cs
- COM2Enum.cs
- TypeBuilderInstantiation.cs
- PreloadedPackages.cs
- DragCompletedEventArgs.cs
- TextViewDesigner.cs
- DataGridViewUtilities.cs
- CompoundFileStorageReference.cs
- StoreItemCollection.Loader.cs
- OracleTimeSpan.cs
- ElementsClipboardData.cs
- RemoveStoryboard.cs
- UnauthorizedWebPart.cs
- CodeLinePragma.cs
- AudioLevelUpdatedEventArgs.cs
- ConfigXmlWhitespace.cs
- DataBindingCollection.cs
- ScrollChangedEventArgs.cs
- SystemEvents.cs
- HatchBrush.cs
- OLEDB_Enum.cs
- LinkedResourceCollection.cs
- ListSortDescription.cs
- SequenceNumber.cs
- DataGrid.cs
- Error.cs
- HttpCacheVaryByContentEncodings.cs
- PerformanceCounterNameAttribute.cs
- HandlerWithFactory.cs
- RegistryPermission.cs
- ControlEvent.cs
- Rules.cs
- DocumentViewerAutomationPeer.cs
- FunctionImportMapping.cs
- RemoteWebConfigurationHostStream.cs
- ToolStripItemTextRenderEventArgs.cs
- TraceSection.cs
- MinimizableAttributeTypeConverter.cs
- SqlCacheDependencyDatabaseCollection.cs
- DescendentsWalker.cs
- HMACSHA1.cs
- UnmanagedMarshal.cs
- SqlExpander.cs
- PenContext.cs
- ResourcePart.cs
- ServiceModelActivity.cs
- StoreContentChangedEventArgs.cs
- ScrollViewer.cs
- FrameDimension.cs
- TCEAdapterGenerator.cs
- XmlCustomFormatter.cs
- DataGridViewCellValidatingEventArgs.cs
- AlgoModule.cs
- BuildDependencySet.cs
- AttributeSetAction.cs
- EventLogException.cs
- BitmapEffectGroup.cs
- TracingConnectionInitiator.cs
- XmlAtomErrorReader.cs
- SQLMoneyStorage.cs
- InvalidWMPVersionException.cs
- WindowsTreeView.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- FocusWithinProperty.cs
- PopOutPanel.cs
- XmlDocumentType.cs
- CTreeGenerator.cs
- ConcurrentBag.cs
- DBCommand.cs
- BooleanStorage.cs
- translator.cs
- HtmlInputImage.cs
- _UriSyntax.cs
- SelectionPattern.cs
- Point.cs
- InputProviderSite.cs
- SafeNativeMethodsCLR.cs
- XdrBuilder.cs
- ValidationHelper.cs
- IListConverters.cs
- Currency.cs
- TypedElement.cs
- StrokeNodeData.cs
- InvokePatternIdentifiers.cs
- PropertyValueChangedEvent.cs
- CodeDefaultValueExpression.cs
- DataGridViewImageCell.cs
- DockAndAnchorLayout.cs