Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / Win32MouseDevice.cs / 1 / Win32MouseDevice.cs
using System.Diagnostics; using System.Collections; using System.Windows; using System.Windows.Media; using System.Windows.Interop; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; // SecurityHelper using MS.Win32; // *NativeMethods using System.Runtime.InteropServices; using System; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// The Win32MouseDevice class implements the platform specific /// MouseDevice features for the Win32 platform /// internal sealed class Win32MouseDevice : MouseDevice { ////// /// /// /// ////// Critical - This is code that elevates AND creates the mouse device which /// happens to hold the callback to filter mouse messages /// TreatAsSafe: This constructor handles critical data but does not expose it /// It stores instance but there are demands on the instances. /// [SecurityCritical,SecurityTreatAsSafe] internal Win32MouseDevice(InputManager inputManager) : base(inputManager) { } ////// Gets the current state of the specified button from the device from the underlying system /// /// /// The mouse button to get the state of /// ////// The state of the specified mouse button /// ////// Critical: Makes calls to UnsafeNativeMethods (GetKeyState) /// TreatAsSafe: Only returns the current state of the specified button /// [SecurityCritical,SecurityTreatAsSafe] internal override MouseButtonState GetButtonStateFromSystem(MouseButton mouseButton) { MouseButtonState mouseButtonState = MouseButtonState.Released; // Security Mitigation: do not give out input state if the device is not active. if(IsActive) { int virtualKeyCode = 0; switch( mouseButton ) { case MouseButton.Left: virtualKeyCode = NativeMethods.VK_LBUTTON; break; case MouseButton.Right: virtualKeyCode = NativeMethods.VK_RBUTTON; break; case MouseButton.Middle: virtualKeyCode = NativeMethods.VK_MBUTTON; break; case MouseButton.XButton1: virtualKeyCode = NativeMethods.VK_XBUTTON1; break; case MouseButton.XButton2: virtualKeyCode = NativeMethods.VK_XBUTTON2; break; } mouseButtonState = ( UnsafeNativeMethods.GetKeyState(virtualKeyCode) & 0x8000 ) != 0 ? MouseButtonState.Pressed : MouseButtonState.Released; } return mouseButtonState; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Diagnostics; using System.Collections; using System.Windows; using System.Windows.Media; using System.Windows.Interop; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; // SecurityHelper using MS.Win32; // *NativeMethods using System.Runtime.InteropServices; using System; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// The Win32MouseDevice class implements the platform specific /// MouseDevice features for the Win32 platform /// internal sealed class Win32MouseDevice : MouseDevice { ////// /// /// /// ////// Critical - This is code that elevates AND creates the mouse device which /// happens to hold the callback to filter mouse messages /// TreatAsSafe: This constructor handles critical data but does not expose it /// It stores instance but there are demands on the instances. /// [SecurityCritical,SecurityTreatAsSafe] internal Win32MouseDevice(InputManager inputManager) : base(inputManager) { } ////// Gets the current state of the specified button from the device from the underlying system /// /// /// The mouse button to get the state of /// ////// The state of the specified mouse button /// ////// Critical: Makes calls to UnsafeNativeMethods (GetKeyState) /// TreatAsSafe: Only returns the current state of the specified button /// [SecurityCritical,SecurityTreatAsSafe] internal override MouseButtonState GetButtonStateFromSystem(MouseButton mouseButton) { MouseButtonState mouseButtonState = MouseButtonState.Released; // Security Mitigation: do not give out input state if the device is not active. if(IsActive) { int virtualKeyCode = 0; switch( mouseButton ) { case MouseButton.Left: virtualKeyCode = NativeMethods.VK_LBUTTON; break; case MouseButton.Right: virtualKeyCode = NativeMethods.VK_RBUTTON; break; case MouseButton.Middle: virtualKeyCode = NativeMethods.VK_MBUTTON; break; case MouseButton.XButton1: virtualKeyCode = NativeMethods.VK_XBUTTON1; break; case MouseButton.XButton2: virtualKeyCode = NativeMethods.VK_XBUTTON2; break; } mouseButtonState = ( UnsafeNativeMethods.GetKeyState(virtualKeyCode) & 0x8000 ) != 0 ? MouseButtonState.Pressed : MouseButtonState.Released; } return mouseButtonState; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
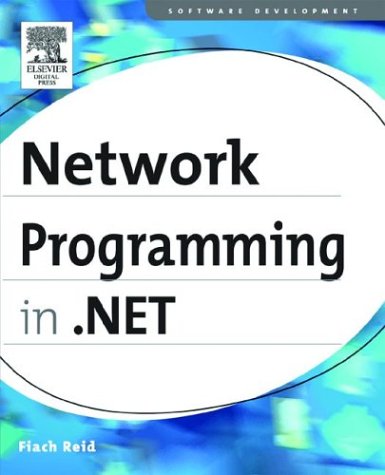
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExpressionBindings.cs
- RoleManagerEventArgs.cs
- MenuItemBinding.cs
- UIAgentCrashedException.cs
- ListSortDescription.cs
- StatusBar.cs
- DelegateArgumentValue.cs
- DesignerDataSchemaClass.cs
- EnumerableRowCollectionExtensions.cs
- ErrorFormatter.cs
- AspNetPartialTrustHelpers.cs
- ServiceOperation.cs
- ClientTargetSection.cs
- PersonalizationStateInfoCollection.cs
- _ScatterGatherBuffers.cs
- BeginStoryboard.cs
- InputScope.cs
- JoinCqlBlock.cs
- HandlerBase.cs
- StringOutput.cs
- LinearKeyFrames.cs
- ActiveXSite.cs
- StatusBarPanelClickEvent.cs
- HtmlTableRowCollection.cs
- PriorityChain.cs
- AlgoModule.cs
- XmlWrappingWriter.cs
- SimpleType.cs
- DataGridViewCheckBoxColumn.cs
- Marshal.cs
- ResXBuildProvider.cs
- DynamicQueryableWrapper.cs
- User.cs
- ServiceControllerDesigner.cs
- BatchServiceHost.cs
- ViewStateException.cs
- WebPartEventArgs.cs
- SingleTagSectionHandler.cs
- PropertyInfoSet.cs
- Events.cs
- SqlDependency.cs
- querybuilder.cs
- ImageIndexEditor.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- XmlQueryTypeFactory.cs
- RawAppCommandInputReport.cs
- WebPartDisplayModeCancelEventArgs.cs
- BitmapEffectrendercontext.cs
- Adorner.cs
- VectorAnimation.cs
- WindowCollection.cs
- CustomAttributeFormatException.cs
- TextProperties.cs
- HttpListenerResponse.cs
- RegexCode.cs
- HttpApplication.cs
- PeerApplication.cs
- XmlDataCollection.cs
- Scene3D.cs
- DbProviderManifest.cs
- TableCellCollection.cs
- ClientSideProviderDescription.cs
- HttpDictionary.cs
- CodeChecksumPragma.cs
- ToolStripDropDownMenu.cs
- RadioButtonBaseAdapter.cs
- Nullable.cs
- TcpActivation.cs
- ListViewCommandEventArgs.cs
- ToolStripItemImageRenderEventArgs.cs
- Trigger.cs
- PropertyGridView.cs
- Number.cs
- RectValueSerializer.cs
- ControlBuilderAttribute.cs
- Italic.cs
- AsymmetricSignatureFormatter.cs
- Parser.cs
- SqlProviderServices.cs
- WebControlsSection.cs
- BaseResourcesBuildProvider.cs
- DesignerActionService.cs
- TrackBarRenderer.cs
- DataGridViewCellStateChangedEventArgs.cs
- XmlDictionaryReader.cs
- HierarchicalDataBoundControl.cs
- InputScope.cs
- TreeBuilder.cs
- ProtocolElement.cs
- FlowPosition.cs
- UnknownBitmapDecoder.cs
- SQLInt32Storage.cs
- TypeConverterHelper.cs
- TextEndOfParagraph.cs
- DecimalAnimationUsingKeyFrames.cs
- StylusPointProperty.cs
- LZCodec.cs
- SplineQuaternionKeyFrame.cs
- DataGridCommandEventArgs.cs
- peernodestatemanager.cs