Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Shared / MS / Internal / ResourceIDHelper.cs / 1 / ResourceIDHelper.cs
using System; using System.Text; using System.Globalization; namespace MS.Internal { internal static class ResourceIDHelper { // // Convert relative path to the right format which is used // as ResourceID. // MarkupCompiler, ResXGen, Loader will call this method internally. // static internal string GetResourceIDFromRelativePath(string relPath) { // It is important that relPath not contain a fragment or query at this point // // Currently this will always be true because // 1) The resources listed in the .proj file are tested against the filesystem // to see if they exist. If a query or fragment was present this test would fail. // 2) In the new loader the container apis make sure that the fragment and query are // stripped from the Uri to form the part name which is used to load the resource Uri baseUri = new Uri("http://foo/"); Uri srcUri = new Uri(baseUri, relPath.Replace("#", "%23")); return GetResourceIDFromUri(baseUri, srcUri); } // // This is the central place that returns right ResourceID for // the passed SourceUri. // static private string GetResourceIDFromUri(Uri baseUri, Uri sourceUri) { string resourceID = String.Empty; // // Extracts and returns the resource ID from a URI. // If the source URI is under the baseUri, the resource ID is the URI // after the baseUri and any fragment or query has been removed. // The resource ID is always converted to lower case. // // If the sourceUri is not relative to baseUri, Emtpy string is returned // as resource id. // if (baseUri.IsAbsoluteUri == false || sourceUri.IsAbsoluteUri == false) { // // if any passed Uri is not absolute uri, return empty string here. // return resourceID; } if (baseUri.Scheme == sourceUri.Scheme && baseUri.Host == sourceUri.Host) { // // Get the escaped Path part, Path doesn't include Query and Fragment. // string basePath = baseUri.GetComponents(UriComponents.Path, UriFormat.UriEscaped); string sourcePath = sourceUri.GetComponents(UriComponents.Path, UriFormat.UriEscaped); // // Always lower case the Path string. // basePath = basePath.ToLower(CultureInfo.InvariantCulture); sourcePath = sourcePath.ToLower(CultureInfo.InvariantCulture); if (sourcePath.StartsWith(basePath, StringComparison.OrdinalIgnoreCase)) { resourceID = sourcePath.Substring(basePath.Length); } } return resourceID; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Text; using System.Globalization; namespace MS.Internal { internal static class ResourceIDHelper { // // Convert relative path to the right format which is used // as ResourceID. // MarkupCompiler, ResXGen, Loader will call this method internally. // static internal string GetResourceIDFromRelativePath(string relPath) { // It is important that relPath not contain a fragment or query at this point // // Currently this will always be true because // 1) The resources listed in the .proj file are tested against the filesystem // to see if they exist. If a query or fragment was present this test would fail. // 2) In the new loader the container apis make sure that the fragment and query are // stripped from the Uri to form the part name which is used to load the resource Uri baseUri = new Uri("http://foo/"); Uri srcUri = new Uri(baseUri, relPath.Replace("#", "%23")); return GetResourceIDFromUri(baseUri, srcUri); } // // This is the central place that returns right ResourceID for // the passed SourceUri. // static private string GetResourceIDFromUri(Uri baseUri, Uri sourceUri) { string resourceID = String.Empty; // // Extracts and returns the resource ID from a URI. // If the source URI is under the baseUri, the resource ID is the URI // after the baseUri and any fragment or query has been removed. // The resource ID is always converted to lower case. // // If the sourceUri is not relative to baseUri, Emtpy string is returned // as resource id. // if (baseUri.IsAbsoluteUri == false || sourceUri.IsAbsoluteUri == false) { // // if any passed Uri is not absolute uri, return empty string here. // return resourceID; } if (baseUri.Scheme == sourceUri.Scheme && baseUri.Host == sourceUri.Host) { // // Get the escaped Path part, Path doesn't include Query and Fragment. // string basePath = baseUri.GetComponents(UriComponents.Path, UriFormat.UriEscaped); string sourcePath = sourceUri.GetComponents(UriComponents.Path, UriFormat.UriEscaped); // // Always lower case the Path string. // basePath = basePath.ToLower(CultureInfo.InvariantCulture); sourcePath = sourcePath.ToLower(CultureInfo.InvariantCulture); if (sourcePath.StartsWith(basePath, StringComparison.OrdinalIgnoreCase)) { resourceID = sourcePath.Substring(basePath.Length); } } return resourceID; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
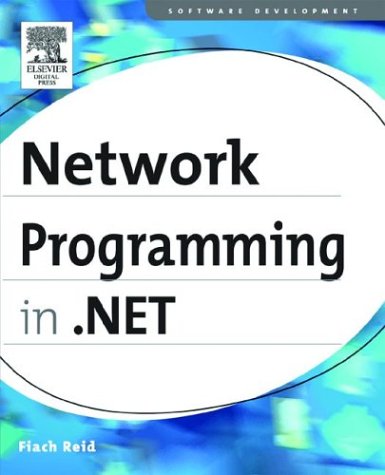
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerNameRecord.cs
- Validator.cs
- ContentDefinition.cs
- ControlBuilderAttribute.cs
- RenderDataDrawingContext.cs
- IgnoreSectionHandler.cs
- SizeF.cs
- ProfileSettings.cs
- HiddenField.cs
- BoolExpression.cs
- CredentialSelector.cs
- NameTable.cs
- AttachedPropertyDescriptor.cs
- ViewSimplifier.cs
- ReaderWriterLock.cs
- ComboBox.cs
- CacheSection.cs
- TraceHandler.cs
- DataViewManagerListItemTypeDescriptor.cs
- ZipPackagePart.cs
- ImpersonationOption.cs
- SingleObjectCollection.cs
- CalculatedColumn.cs
- DataColumnPropertyDescriptor.cs
- DelayedRegex.cs
- XmlSchemaSimpleTypeRestriction.cs
- ManagementObject.cs
- SignatureDescription.cs
- Int16Converter.cs
- WebPartsPersonalizationAuthorization.cs
- DashStyles.cs
- TextCollapsingProperties.cs
- SplineKeyFrames.cs
- WizardPanel.cs
- SingleObjectCollection.cs
- DataGrid.cs
- Application.cs
- ProfileEventArgs.cs
- DateTimeFormatInfo.cs
- MultilineStringConverter.cs
- CursorEditor.cs
- RenderData.cs
- IUnknownConstantAttribute.cs
- RemotingService.cs
- IntPtr.cs
- ScriptDescriptor.cs
- TextRenderer.cs
- FileUpload.cs
- NetPeerTcpBindingElement.cs
- storepermission.cs
- QueueProcessor.cs
- JavaScriptSerializer.cs
- MaterialCollection.cs
- Constants.cs
- CompilerResults.cs
- RtfControlWordInfo.cs
- PeerNodeTraceRecord.cs
- RPIdentityRequirement.cs
- WorkflowItemsPresenter.cs
- DataContractSet.cs
- Touch.cs
- Canvas.cs
- NavigationWindow.cs
- XXXInfos.cs
- SafeNativeMethods.cs
- GenericRootAutomationPeer.cs
- ClassicBorderDecorator.cs
- FilteredDataSetHelper.cs
- ContentPropertyAttribute.cs
- CodeSnippetCompileUnit.cs
- DiscardableAttribute.cs
- Listbox.cs
- SolidBrush.cs
- GetKeyedHashRequest.cs
- WindowsFormsHost.cs
- MenuBase.cs
- TargetFrameworkAttribute.cs
- JulianCalendar.cs
- DirectoryNotFoundException.cs
- EntityProviderFactory.cs
- ExpressionPrefixAttribute.cs
- TrackBar.cs
- SchemaHelper.cs
- CommandBinding.cs
- DataGridViewLayoutData.cs
- CodeAssignStatement.cs
- Soap12ProtocolImporter.cs
- PingOptions.cs
- OpenTypeCommon.cs
- NotifyParentPropertyAttribute.cs
- InputLanguage.cs
- _UncName.cs
- ConfigurationFileMap.cs
- Timer.cs
- RootContext.cs
- DataMember.cs
- XsltConvert.cs
- XPathDocumentNavigator.cs
- CaseStatementProjectedSlot.cs
- QilCloneVisitor.cs