Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Shared / MS / Internal / WeakReferenceEnumerator.cs / 1 / WeakReferenceEnumerator.cs
using System; using System.Collections; using System.Windows; #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif // Disable pragma warnings to enable PREsharp pragmas #pragma warning disable 1634, 1691 namespace MS.Internal { ////// This allows callers to "foreach" through a WeakReferenceList. /// Each weakreference is checked for liveness and "current" /// actually returns a strong reference to the current element. /// ////// Due to the way enumerators function, this enumerator often /// holds a cached strong reference to the "Current" element. /// This should not be a problem unless the caller stops enumerating /// before the end of the list AND holds the enumerator alive forever. /// [FriendAccessAllowed] internal struct WeakReferenceListEnumerator : IEnumerator { public WeakReferenceListEnumerator( ArrayList List) { _i = 0; _List = List; _StrongReference = null; } object IEnumerator.Current { get{ return Current; } } public object Current { get { if( null == _StrongReference ) { #pragma warning suppress 6503 throw new System.InvalidOperationException(SR.Get(SRID.Enumerator_VerifyContext)); } return _StrongReference; } } public bool MoveNext() { object obj=null; while( _i < _List.Count ) { WeakReference weakRef = (WeakReference) _List[ _i++ ]; obj = weakRef.Target; if(null != obj) break; } _StrongReference = obj; return (null != obj); } public void Reset() { _i = 0; _StrongReference = null; } int _i; ArrayList _List; object _StrongReference; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Windows; #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif // Disable pragma warnings to enable PREsharp pragmas #pragma warning disable 1634, 1691 namespace MS.Internal { ////// This allows callers to "foreach" through a WeakReferenceList. /// Each weakreference is checked for liveness and "current" /// actually returns a strong reference to the current element. /// ////// Due to the way enumerators function, this enumerator often /// holds a cached strong reference to the "Current" element. /// This should not be a problem unless the caller stops enumerating /// before the end of the list AND holds the enumerator alive forever. /// [FriendAccessAllowed] internal struct WeakReferenceListEnumerator : IEnumerator { public WeakReferenceListEnumerator( ArrayList List) { _i = 0; _List = List; _StrongReference = null; } object IEnumerator.Current { get{ return Current; } } public object Current { get { if( null == _StrongReference ) { #pragma warning suppress 6503 throw new System.InvalidOperationException(SR.Get(SRID.Enumerator_VerifyContext)); } return _StrongReference; } } public bool MoveNext() { object obj=null; while( _i < _List.Count ) { WeakReference weakRef = (WeakReference) _List[ _i++ ]; obj = weakRef.Target; if(null != obj) break; } _StrongReference = obj; return (null != obj); } public void Reset() { _i = 0; _StrongReference = null; } int _i; ArrayList _List; object _StrongReference; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
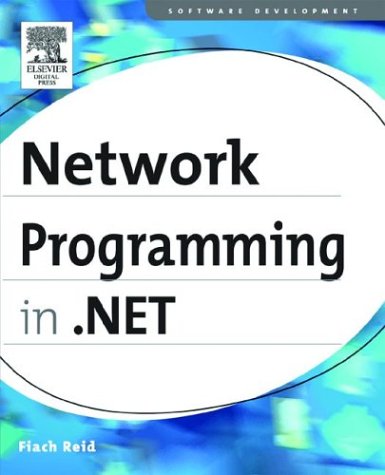
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ContentElementAutomationPeer.cs
- AffineTransform3D.cs
- GenericEnumerator.cs
- TargetControlTypeAttribute.cs
- AdornerHitTestResult.cs
- PageBreakRecord.cs
- PrintingPermission.cs
- keycontainerpermission.cs
- StackSpiller.cs
- LassoHelper.cs
- Opcode.cs
- EndpointDiscoveryMetadataCD1.cs
- BooleanStorage.cs
- AutomationPropertyInfo.cs
- CustomErrorsSection.cs
- ProviderIncompatibleException.cs
- EndpointBehaviorElement.cs
- BitmapPalette.cs
- DbDeleteCommandTree.cs
- MSAANativeProvider.cs
- TextServicesContext.cs
- CommandHelper.cs
- CustomCategoryAttribute.cs
- BulletedListEventArgs.cs
- WindowsIPAddress.cs
- MetadataHelper.cs
- MenuCommand.cs
- GridViewSelectEventArgs.cs
- AliasedSlot.cs
- XslTransformFileEditor.cs
- NameValuePair.cs
- TextViewBase.cs
- PersonalizationAdministration.cs
- SelectionRangeConverter.cs
- Bezier.cs
- EdmFunctions.cs
- StaticExtensionConverter.cs
- DataRecordObjectView.cs
- ApplicationManager.cs
- BitSet.cs
- ConfigurationSchemaErrors.cs
- ColorMap.cs
- TreeViewImageKeyConverter.cs
- LoadItemsEventArgs.cs
- XmlElementCollection.cs
- ComplexObject.cs
- CriticalFinalizerObject.cs
- StrokeFIndices.cs
- CaseCqlBlock.cs
- CompilerErrorCollection.cs
- LayoutEngine.cs
- TableHeaderCell.cs
- TemplateField.cs
- HMACSHA1.cs
- DataGridPageChangedEventArgs.cs
- PropertyInformation.cs
- RegistryPermission.cs
- ActivityInstance.cs
- MemberCollection.cs
- QueryResult.cs
- XmlSequenceWriter.cs
- HostingEnvironmentSection.cs
- BinaryObjectInfo.cs
- iisPickupDirectory.cs
- SortDescriptionCollection.cs
- SkewTransform.cs
- TableRow.cs
- DataColumnCollection.cs
- AttributeCallbackBuilder.cs
- StringWriter.cs
- DbConnectionPoolGroupProviderInfo.cs
- WindowsListViewScroll.cs
- PasswordTextNavigator.cs
- PrintDialogException.cs
- XmlAnyAttributeAttribute.cs
- ContentValidator.cs
- InputProviderSite.cs
- Baml2006ReaderContext.cs
- EntityException.cs
- ExceptionUtil.cs
- NegotiateStream.cs
- SQLGuid.cs
- ExpressionEvaluator.cs
- RegisteredDisposeScript.cs
- TextEffect.cs
- MsmqIntegrationSecurityMode.cs
- SafeNativeMethods.cs
- EntityProviderServices.cs
- RankException.cs
- DbInsertCommandTree.cs
- MarshalByRefObject.cs
- SerialErrors.cs
- PictureBox.cs
- EventWaitHandleSecurity.cs
- WebConfigurationHostFileChange.cs
- BasicViewGenerator.cs
- FixedFlowMap.cs
- HttpRequestBase.cs
- TokenBasedSet.cs
- OptimalBreakSession.cs