Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / BuildProvider.cs / 2 / BuildProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Compilation; using System.Reflection; using System.Web.Hosting; using System.Web.UI; using System.CodeDom.Compiler; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class BuildProvider : ConfigurationElement { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propExtension = new ConfigurationProperty("extension", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propType = new ConfigurationProperty("type", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); private Type _type; // AppliesTo value from the BuildProviderAppliesToAttribute private BuildProviderAppliesTo _appliesToInternal; static BuildProvider() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propExtension); _properties.Add(_propType); } public BuildProvider(String extension, String type) : this() { Extension = extension; Type = type; } internal BuildProvider() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } // this override is required because AppliesTo may be in any order in the // property string but it still and the default equals operator would public override bool Equals(object provider) { BuildProvider o = provider as BuildProvider; return (o != null && StringUtil.EqualsIgnoreCase(Extension, o.Extension) && Type == o.Type); } public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(Extension.ToLower(CultureInfo.InvariantCulture).GetHashCode(), Type.GetHashCode()); } [ConfigurationProperty("extension", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Extension { get { return (string)base[_propExtension]; } set { base[_propExtension] = value; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Type { get { return (string)base[_propType]; } set { base[_propType] = value; } } internal Type TypeInternal { get { if (_type == null) { lock (this) { if (_type == null) { _type = CompilationUtil.LoadTypeWithChecks(Type, typeof(System.Web.Compilation.BuildProvider), null, this, "type"); } } } return _type; } } internal BuildProviderAppliesTo AppliesToInternal { get { if (_appliesToInternal != 0) return _appliesToInternal; // Check whether the control builder's class exposes an AppliesTo attribute object[] attrs = TypeInternal.GetCustomAttributes( typeof(BuildProviderAppliesToAttribute), /*inherit*/ true); if ((attrs != null) && (attrs.Length > 0)) { Debug.Assert(attrs[0] is BuildProviderAppliesToAttribute); _appliesToInternal = ((BuildProviderAppliesToAttribute)attrs[0]).AppliesTo; } else { // Default to applying to All _appliesToInternal = BuildProviderAppliesTo.All; } return _appliesToInternal; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Compilation; using System.Reflection; using System.Web.Hosting; using System.Web.UI; using System.CodeDom.Compiler; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class BuildProvider : ConfigurationElement { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propExtension = new ConfigurationProperty("extension", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propType = new ConfigurationProperty("type", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); private Type _type; // AppliesTo value from the BuildProviderAppliesToAttribute private BuildProviderAppliesTo _appliesToInternal; static BuildProvider() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propExtension); _properties.Add(_propType); } public BuildProvider(String extension, String type) : this() { Extension = extension; Type = type; } internal BuildProvider() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } // this override is required because AppliesTo may be in any order in the // property string but it still and the default equals operator would public override bool Equals(object provider) { BuildProvider o = provider as BuildProvider; return (o != null && StringUtil.EqualsIgnoreCase(Extension, o.Extension) && Type == o.Type); } public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(Extension.ToLower(CultureInfo.InvariantCulture).GetHashCode(), Type.GetHashCode()); } [ConfigurationProperty("extension", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Extension { get { return (string)base[_propExtension]; } set { base[_propExtension] = value; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Type { get { return (string)base[_propType]; } set { base[_propType] = value; } } internal Type TypeInternal { get { if (_type == null) { lock (this) { if (_type == null) { _type = CompilationUtil.LoadTypeWithChecks(Type, typeof(System.Web.Compilation.BuildProvider), null, this, "type"); } } } return _type; } } internal BuildProviderAppliesTo AppliesToInternal { get { if (_appliesToInternal != 0) return _appliesToInternal; // Check whether the control builder's class exposes an AppliesTo attribute object[] attrs = TypeInternal.GetCustomAttributes( typeof(BuildProviderAppliesToAttribute), /*inherit*/ true); if ((attrs != null) && (attrs.Length > 0)) { Debug.Assert(attrs[0] is BuildProviderAppliesToAttribute); _appliesToInternal = ((BuildProviderAppliesToAttribute)attrs[0]).AppliesTo; } else { // Default to applying to All _appliesToInternal = BuildProviderAppliesTo.All; } return _appliesToInternal; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
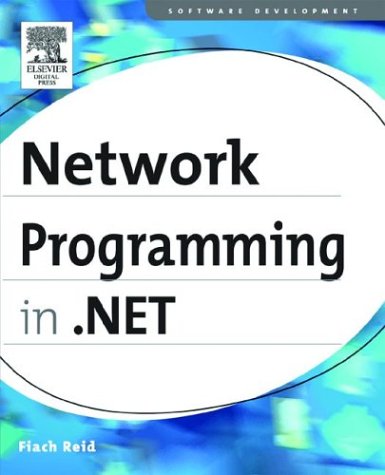
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsTooltip.cs
- XmlSchemaCompilationSettings.cs
- ToolboxItem.cs
- X509ServiceCertificateAuthenticationElement.cs
- _LocalDataStore.cs
- SafeEventLogWriteHandle.cs
- ReachPrintTicketSerializer.cs
- DataGridViewHitTestInfo.cs
- XmlDataDocument.cs
- InitializerFacet.cs
- WizardSideBarListControlItem.cs
- DataGridBoolColumn.cs
- XmlNullResolver.cs
- Msec.cs
- CallInfo.cs
- GetWinFXPath.cs
- SQlBooleanStorage.cs
- PermissionToken.cs
- TextRange.cs
- _Connection.cs
- ScriptReferenceEventArgs.cs
- PhysicalFontFamily.cs
- ConfigXmlSignificantWhitespace.cs
- CodeDOMProvider.cs
- ObjectTokenCategory.cs
- ToolStripPanelSelectionGlyph.cs
- CookieParameter.cs
- ComplexType.cs
- XmlLanguageConverter.cs
- EntityDataSourceReferenceGroup.cs
- DefaultObjectSerializer.cs
- CommandConverter.cs
- CallSite.cs
- DbDataAdapter.cs
- PenCursorManager.cs
- Vector3DCollection.cs
- TextModifierScope.cs
- NodeLabelEditEvent.cs
- TableCellAutomationPeer.cs
- Quad.cs
- ErrorTableItemStyle.cs
- SocketAddress.cs
- AstNode.cs
- SystemIPInterfaceStatistics.cs
- GlobalizationSection.cs
- ItemsPanelTemplate.cs
- CutCopyPasteHelper.cs
- PropertyEntry.cs
- AccessibilityHelperForVista.cs
- StaticTextPointer.cs
- Random.cs
- CodePageEncoding.cs
- PageTheme.cs
- ProcessModuleCollection.cs
- AsyncPostBackErrorEventArgs.cs
- ExpressionTextBox.xaml.cs
- TailCallAnalyzer.cs
- VisualBrush.cs
- DbConnectionPool.cs
- RuleProcessor.cs
- CheckBoxRenderer.cs
- FormsIdentity.cs
- LocationUpdates.cs
- DelegatingTypeDescriptionProvider.cs
- JsonSerializer.cs
- InternalException.cs
- PropertyIDSet.cs
- BufferedGraphicsManager.cs
- DBAsyncResult.cs
- ImageIndexConverter.cs
- Utilities.cs
- XmlNavigatorStack.cs
- HtmlShim.cs
- MediaContextNotificationWindow.cs
- DataGridViewColumn.cs
- TextEndOfSegment.cs
- ObjectListItem.cs
- StreamResourceInfo.cs
- OleDbDataAdapter.cs
- DbParameterCollection.cs
- ReadWriteObjectLock.cs
- SmiRecordBuffer.cs
- Validator.cs
- KeyFrames.cs
- ConsoleTraceListener.cs
- HandlerMappingMemo.cs
- MethodAccessException.cs
- FtpRequestCacheValidator.cs
- PolicyLevel.cs
- RewritingValidator.cs
- IItemProperties.cs
- OletxVolatileEnlistment.cs
- ConfigurationManagerHelperFactory.cs
- Configuration.cs
- User.cs
- UntrustedRecipientException.cs
- ListenerUnsafeNativeMethods.cs
- TypeSystemProvider.cs
- Brush.cs
- ListenerSingletonConnectionReader.cs