Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / QueryMath.cs / 1 / QueryMath.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System.Collections; using System.Diagnostics; internal enum MathOperator { None, Plus, Minus, Div, Multiply, Mod, Negate } internal class MathOpcode : Opcode { MathOperator mathOp; internal MathOpcode(OpcodeID id, MathOperator op) : base(id) { this.mathOp = op; } internal override bool Equals(Opcode op) { if (base.Equals(op)) { return (this.mathOp == ((MathOpcode) op).mathOp); } return false; } #if DEBUG_FILTER public override string ToString() { return string.Format("{0} {1}", base.ToString(), this.mathOp.ToString()); } #endif } internal class PlusOpcode : MathOpcode { internal PlusOpcode() : base(OpcodeID.Plus, MathOperator.Plus) { } internal override Opcode Eval(ProcessingContext context) { StackFrame argX = context.TopArg; StackFrame argY = context.SecondArg; DiagnosticUtility.DebugAssert(argX.Count == argY.Count, ""); Value[] values = context.Values; for (int x = argX.basePtr, y = argY.basePtr; x <= argX.endPtr; ++x, ++y) { DiagnosticUtility.DebugAssert(values[x].IsType(ValueDataType.Double), ""); DiagnosticUtility.DebugAssert(values[y].IsType(ValueDataType.Double), ""); values[y].Add(values[x].Double); } context.PopFrame(); return this.next; } } internal class MinusOpcode : MathOpcode { internal MinusOpcode() : base(OpcodeID.Minus, MathOperator.Minus) { } internal override Opcode Eval(ProcessingContext context) { StackFrame argX = context.TopArg; StackFrame argY = context.SecondArg; DiagnosticUtility.DebugAssert(argX.Count == argY.Count, ""); Value[] values = context.Values; for (int x = argX.basePtr, y = argY.basePtr; x <= argX.endPtr; ++x, ++y) { DiagnosticUtility.DebugAssert(values[x].IsType(ValueDataType.Double), ""); DiagnosticUtility.DebugAssert(values[y].IsType(ValueDataType.Double), ""); values[y].Double = values[x].Double - values[y].Double; } context.PopFrame(); return this.next; } } internal class MultiplyOpcode : MathOpcode { internal MultiplyOpcode() : base(OpcodeID.Multiply, MathOperator.Multiply) { } internal override Opcode Eval(ProcessingContext context) { StackFrame argX = context.TopArg; StackFrame argY = context.SecondArg; DiagnosticUtility.DebugAssert(argX.Count == argY.Count, ""); Value[] values = context.Values; for (int x = argX.basePtr, y = argY.basePtr; x <= argX.endPtr; ++x, ++y) { DiagnosticUtility.DebugAssert(values[x].IsType(ValueDataType.Double), ""); DiagnosticUtility.DebugAssert(values[y].IsType(ValueDataType.Double), ""); values[y].Multiply(values[x].Double); } context.PopFrame(); return this.next; } } internal class DivideOpcode : MathOpcode { internal DivideOpcode() : base(OpcodeID.Divide, MathOperator.Div) { } internal override Opcode Eval(ProcessingContext context) { StackFrame argX = context.TopArg; StackFrame argY = context.SecondArg; DiagnosticUtility.DebugAssert(argX.Count == argY.Count, ""); Value[] values = context.Values; for (int x = argX.basePtr, y = argY.basePtr; x <= argX.endPtr; ++x, ++y) { DiagnosticUtility.DebugAssert(values[x].IsType(ValueDataType.Double), ""); DiagnosticUtility.DebugAssert(values[y].IsType(ValueDataType.Double), ""); values[y].Double = values[x].Double / values[y].Double; } context.PopFrame(); return this.next; } } internal class ModulusOpcode : MathOpcode { internal ModulusOpcode() : base(OpcodeID.Mod, MathOperator.Mod) { } internal override Opcode Eval(ProcessingContext context) { StackFrame argX = context.TopArg; StackFrame argY = context.SecondArg; Value[] values = context.Values; DiagnosticUtility.DebugAssert(argX.Count == argY.Count, ""); for (int x = argX.basePtr, y = argY.basePtr; x <= argX.endPtr; ++x, ++y) { DiagnosticUtility.DebugAssert(values[x].IsType(ValueDataType.Double), ""); DiagnosticUtility.DebugAssert(values[y].IsType(ValueDataType.Double), ""); values[y].Double = values[x].Double % values[y].Double; } context.PopFrame(); return this.next; } } internal class NegateOpcode : MathOpcode { internal NegateOpcode() : base(OpcodeID.Negate, MathOperator.Negate) { } internal override Opcode Eval(ProcessingContext context) { StackFrame frame = context.TopArg; Value[] values = context.Values; for (int i = frame.basePtr; i <= frame.endPtr; ++i) { values[i].Negate(); } return this.next; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
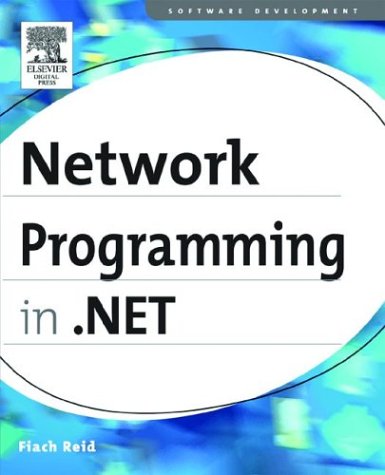
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DateTime.cs
- ImportCatalogPart.cs
- UncommonField.cs
- MenuItem.cs
- AbsoluteQuery.cs
- HtmlWindowCollection.cs
- SmtpCommands.cs
- RegexInterpreter.cs
- initElementDictionary.cs
- AspNetHostingPermission.cs
- BitmapEffect.cs
- RouteParametersHelper.cs
- FaultContractAttribute.cs
- SqlBooleanMismatchVisitor.cs
- ScrollEventArgs.cs
- ISAPIRuntime.cs
- AspNetPartialTrustHelpers.cs
- OdbcException.cs
- SQLBinaryStorage.cs
- TextContainerChangedEventArgs.cs
- Bidi.cs
- CounterCreationDataCollection.cs
- Storyboard.cs
- CodeGenerator.cs
- XmlWriter.cs
- ToolBar.cs
- VoiceObjectToken.cs
- HuffModule.cs
- ObjectDataSourceSelectingEventArgs.cs
- ProcessProtocolHandler.cs
- SqlLiftWhereClauses.cs
- Rectangle.cs
- GuidelineCollection.cs
- ImageAutomationPeer.cs
- SecurityToken.cs
- XmlSerializableWriter.cs
- CheckBox.cs
- XmlConvert.cs
- DataSourceUtil.cs
- FunctionImportMapping.ReturnTypeRenameMapping.cs
- XmlSchemas.cs
- EncoderFallback.cs
- ValidatingReaderNodeData.cs
- MonitoringDescriptionAttribute.cs
- ListBoxItem.cs
- FormClosedEvent.cs
- DateTimeUtil.cs
- ControlSerializer.cs
- SvcFileManager.cs
- BindingContext.cs
- ExtractorMetadata.cs
- GeometryModel3D.cs
- ADMembershipProvider.cs
- SourceFileInfo.cs
- LinearQuaternionKeyFrame.cs
- BasicCellRelation.cs
- FontFaceLayoutInfo.cs
- IProvider.cs
- TransportOutputChannel.cs
- DecoderFallbackWithFailureFlag.cs
- NavigatingCancelEventArgs.cs
- DelimitedListTraceListener.cs
- ArgumentException.cs
- SqlDataSource.cs
- DataGridViewRowsRemovedEventArgs.cs
- DataColumnSelectionConverter.cs
- RouteUrlExpressionBuilder.cs
- Rotation3D.cs
- SystemIcmpV6Statistics.cs
- AxisAngleRotation3D.cs
- AxHost.cs
- DataGridPagerStyle.cs
- Overlapped.cs
- ThicknessConverter.cs
- HttpProfileBase.cs
- ResourceProperty.cs
- LoginName.cs
- NoPersistHandle.cs
- ExpressionCopier.cs
- StrokeNodeEnumerator.cs
- PasswordBoxAutomationPeer.cs
- XmlHierarchicalEnumerable.cs
- XmlSchemas.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- ClientTargetCollection.cs
- DataSourceHelper.cs
- OdbcHandle.cs
- Bidi.cs
- MessageEncodingBindingElementImporter.cs
- IPAddress.cs
- TeredoHelper.cs
- XmlIgnoreAttribute.cs
- SystemIcmpV6Statistics.cs
- ExpandSegmentCollection.cs
- SizeChangedInfo.cs
- UndoEngine.cs
- X509SecurityToken.cs
- FastEncoderWindow.cs
- SiteMapDataSourceView.cs
- XPathConvert.cs