Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / Behavior / Behavior.cs / 1 / Behavior.cs
namespace System.Windows.Forms.Design.Behavior { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Windows.Forms.Design; ////// /// This abstract class represents the Behavior objects that are managed /// by the BehaviorService. This class can be extended to develop any /// type of UI 'behavior'. Ex: selection, drag, and resize behaviors. /// [SuppressMessage("Microsoft.Naming", "CA1724:TypeNamesShouldNotMatchNamespaces")] public abstract class Behavior { private bool callParentBehavior = false; private BehaviorService bhvSvc; protected Behavior() { } ////// /// /// callParentBehavior - true if the parentBehavior should be called if it exists. The /// parentBehavior is the next behavior on the behaviorService stack. /// /// If callParentBehavior is true, then behaviorService must be non-null /// /// protected Behavior(bool callParentBehavior, BehaviorService behaviorService) { if ((callParentBehavior == true) && (behaviorService == null)) { throw new ArgumentException("behaviorService"); } this.callParentBehavior = callParentBehavior; this.bhvSvc = behaviorService; } private Behavior GetNextBehavior { get { if (bhvSvc != null) { return bhvSvc.GetNextBehavior(this); } return null; } } ////// /// The cursor that should be displayed for this behavior. /// public virtual Cursor Cursor { get { return Cursors.Default; } } ////// /// Rerturning true from here indicates to the BehaviorService that /// all MenuCommands the designer receives should have their /// state set to 'Enabled = false' when this Behavior is active. /// public virtual bool DisableAllCommands { get { if(callParentBehavior && GetNextBehavior != null) { return GetNextBehavior.DisableAllCommands; } else { return false; } } } ////// /// Called from the BehaviorService, this function provides an opportunity /// for the Behavior to return its own custom MenuCommand thereby /// intercepting this message. /// [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes")] [SuppressMessage("Microsoft.Security", "CA2102:CatchNonClsCompliantExceptionsInGeneralHandlers")] public virtual MenuCommand FindCommand(CommandID commandId) { try { if(callParentBehavior && GetNextBehavior != null) { return GetNextBehavior.FindCommand(commandId); } else { return null; } } catch //Catch any exception and return null MenuCommand. { return null; } } ////// The heuristic we will follow when any of these methods are called /// is that we will attempt to pass the message along to the glyph. /// This is a helper method to ensure validity before forwarding the message. /// private bool GlyphIsValid(Glyph g) { return g != null && g.Behavior != null && g.Behavior != this; } ////// /// A behavior can request mouse capture through the behavior service by pushing /// itself with PushCaptureBehavior. If it does so, it will be notified through /// OnLoseCapture when capture is lost. Generally the behavior pops itself at /// this time. Capture is lost when one of the following occurs: /// /// 1. Someone else requests capture. /// 2. Another behavior is pushed. /// 3. This behavior is popped. /// /// In each of these cases OnLoseCapture on the behavior will be called. /// public virtual void OnLoseCapture(Glyph g, EventArgs e) { if(callParentBehavior && GetNextBehavior != null) { GetNextBehavior.OnLoseCapture(g, e); } else if (GlyphIsValid(g)) { g.Behavior.OnLoseCapture(g, e); } } ////// /// When any MouseDown message enters the BehaviorService's AdornerWindow /// (nclbuttondown, lbuttondown, rbuttondown, nclrbuttondown) it is first /// passed here, to the top-most Behavior in the BehaviorStack. Returning /// 'true' from this function signifies that the Message was 'handled' by /// the Behavior and should not continue to be processed. /// public virtual bool OnMouseDoubleClick(Glyph g, MouseButtons button, Point mouseLoc) { if(callParentBehavior && GetNextBehavior != null) { return GetNextBehavior.OnMouseDoubleClick(g, button, mouseLoc); } else if (GlyphIsValid(g)) { return g.Behavior.OnMouseDoubleClick(g, button, mouseLoc); } else { return false; } } ////// /// When any MouseDown message enters the BehaviorService's AdornerWindow /// (nclbuttondown, lbuttondown, rbuttondown, nclrbuttondown) it is first /// passed here, to the top-most Behavior in the BehaviorStack. Returning /// 'true' from this function signifies that the Message was 'handled' by /// the Behavior and should not continue to be processed. /// public virtual bool OnMouseDown(Glyph g, MouseButtons button, Point mouseLoc) { if(callParentBehavior && GetNextBehavior != null) { return GetNextBehavior.OnMouseDown(g, button, mouseLoc); } else if (GlyphIsValid(g)) { return g.Behavior.OnMouseDown(g, button, mouseLoc); } else { return false; } } ////// /// When the mouse pointer's location is positively hit-tested with a /// different Glyph than previous hit-tests, this event is fired on the /// Behavior associated with the Glyph. /// public virtual bool OnMouseEnter(Glyph g) { if(callParentBehavior && GetNextBehavior != null) { return GetNextBehavior.OnMouseEnter(g); } else if (GlyphIsValid(g)) { return g.Behavior.OnMouseEnter(g); } else { return false; } } ////// /// When a MouseHover message enters the BehaviorService's AdornerWindow /// it is first passed here, to the top-most Behavior /// in the BehaviorStack. Returning 'true' from this function signifies that /// the Message was 'handled' by the Behavior and should not continue to be processed. /// public virtual bool OnMouseHover(Glyph g, Point mouseLoc) { if(callParentBehavior && GetNextBehavior != null) { return GetNextBehavior.OnMouseHover(g, mouseLoc); } else if (GlyphIsValid(g)) { return g.Behavior.OnMouseHover(g, mouseLoc); } else { return false; } } ////// /// When the mouse pointer leaves a positively hit-tested Glyph /// with a valid Behavior, this method is invoked. /// public virtual bool OnMouseLeave(Glyph g) { if(callParentBehavior && GetNextBehavior != null) { return GetNextBehavior.OnMouseLeave(g); } else if (GlyphIsValid(g)) { return g.Behavior.OnMouseLeave(g); } else { return false; } } ////// /// When any MouseMove message enters the BehaviorService's AdornerWindow /// (mousemove, ncmousemove) it is first passed here, to the top-most Behavior /// in the BehaviorStack. Returning 'true' from this function signifies that /// the Message was 'handled' by the Behavior and should not continue to be processed. /// public virtual bool OnMouseMove(Glyph g, MouseButtons button, Point mouseLoc) { if(callParentBehavior && GetNextBehavior != null) { return GetNextBehavior.OnMouseMove(g, button, mouseLoc); } else if (GlyphIsValid(g)) { return g.Behavior.OnMouseMove(g, button, mouseLoc); } else { return false; } } ////// /// When any MouseUp message enters the BehaviorService's AdornerWindow /// (nclbuttonupown, lbuttonup, rbuttonup, nclrbuttonup) it is first /// passed here, to the top-most Behavior in the BehaviorStack. Returning /// 'true' from this function signifies that the Message was 'handled' by /// the Behavior and should not continue to be processed. /// public virtual bool OnMouseUp(Glyph g, MouseButtons button) { if(callParentBehavior && GetNextBehavior != null) { return GetNextBehavior.OnMouseUp(g, button); } else if (GlyphIsValid(g)) { return g.Behavior.OnMouseUp(g, button); } else { return false; } } //OLE DragDrop virtual methods // ////// /// OnDragDrop can be overridden so that a Behavior can specify its own /// Drag/Drop rules. /// public virtual void OnDragDrop(Glyph g, DragEventArgs e) { if(callParentBehavior && GetNextBehavior != null) { GetNextBehavior.OnDragDrop(g, e); } else if (GlyphIsValid(g)) { g.Behavior.OnDragDrop(g, e); } } ////// /// OnDragEnter can be overridden so that a Behavior can specify its own /// Drag/Drop rules. /// public virtual void OnDragEnter(Glyph g, DragEventArgs e) { if(callParentBehavior && GetNextBehavior != null) { GetNextBehavior.OnDragEnter(g, e); } else if (GlyphIsValid(g)) { g.Behavior.OnDragEnter(g, e); } } ////// /// OnDragLeave can be overridden so that a Behavior can specify its own /// Drag/Drop rules. /// public virtual void OnDragLeave(Glyph g, EventArgs e) { if(callParentBehavior && GetNextBehavior != null) { GetNextBehavior.OnDragLeave(g, e); } else if (GlyphIsValid(g)) { g.Behavior.OnDragLeave(g, e); } } ////// /// OnDragOver can be overridden so that a Behavior can specify its own /// Drag/Drop rules. /// public virtual void OnDragOver(Glyph g, DragEventArgs e) { if(callParentBehavior && GetNextBehavior != null) { GetNextBehavior.OnDragOver(g, e); } else if (GlyphIsValid(g)) { g.Behavior.OnDragOver(g, e); } else if (e.Effect != DragDropEffects.None) { e.Effect = (Control.ModifierKeys == Keys.Control) ? DragDropEffects.Copy : DragDropEffects.Move; } } ////// /// OnGiveFeedback can be overridden so that a Behavior can specify its own /// Drag/Drop rules. /// public virtual void OnGiveFeedback(Glyph g, GiveFeedbackEventArgs e) { if(callParentBehavior && GetNextBehavior != null) { GetNextBehavior.OnGiveFeedback(g, e); } else if (GlyphIsValid(g)) { g.Behavior.OnGiveFeedback(g, e); } } ////// /// QueryContinueDrag can be overridden so that a Behavior can specify its own /// Drag/Drop rules. /// public virtual void OnQueryContinueDrag(Glyph g, QueryContinueDragEventArgs e) { if(callParentBehavior && GetNextBehavior != null) { GetNextBehavior.OnQueryContinueDrag(g, e); } else if (GlyphIsValid(g)) { g.Behavior.OnQueryContinueDrag(g, e); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
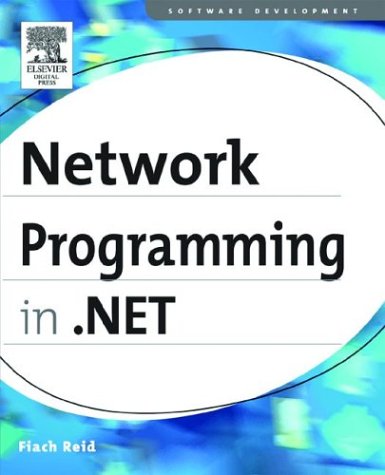
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LicenseManager.cs
- UIElementCollection.cs
- AsyncDataRequest.cs
- RotateTransform.cs
- ChildTable.cs
- IdnElement.cs
- FaultCode.cs
- WebPartRestoreVerb.cs
- PieceDirectory.cs
- PageContentCollection.cs
- XamlRtfConverter.cs
- GeneralTransform3DTo2D.cs
- GridSplitter.cs
- TextBoxDesigner.cs
- TypeReference.cs
- AsyncResult.cs
- InkCollectionBehavior.cs
- SnapshotChangeTrackingStrategy.cs
- DependencyPropertyConverter.cs
- EventTrigger.cs
- SingleSelectRootGridEntry.cs
- ContentPresenter.cs
- Argument.cs
- AssemblyInfo.cs
- TransformedBitmap.cs
- ApplicationFileParser.cs
- metadatamappinghashervisitor.hashsourcebuilder.cs
- ListViewItem.cs
- StylusButton.cs
- EdmSchemaAttribute.cs
- Hash.cs
- ValueUtilsSmi.cs
- DateTimeConverter.cs
- TraceFilter.cs
- ObsoleteAttribute.cs
- GetCertificateRequest.cs
- CacheForPrimitiveTypes.cs
- CryptoStream.cs
- ModelFactory.cs
- RotateTransform.cs
- NetPipeSectionData.cs
- LogManagementAsyncResult.cs
- ResourcePool.cs
- ScriptingWebServicesSectionGroup.cs
- PipelineModuleStepContainer.cs
- PropertyDescriptorGridEntry.cs
- sqlnorm.cs
- XPathPatternParser.cs
- ReaderWriterLock.cs
- Container.cs
- Light.cs
- TextStore.cs
- ListBase.cs
- EdmValidator.cs
- WebPartHelpVerb.cs
- TransformValueSerializer.cs
- VersionedStream.cs
- FieldMetadata.cs
- XMLUtil.cs
- MemoryStream.cs
- BreakRecordTable.cs
- CompareValidator.cs
- PackUriHelper.cs
- PasswordDeriveBytes.cs
- ADRoleFactoryConfiguration.cs
- ToolStripDesignerAvailabilityAttribute.cs
- SqlStatistics.cs
- TransformedBitmap.cs
- UnmanagedHandle.cs
- CustomAssemblyResolver.cs
- X509Certificate2.cs
- CardSpaceSelector.cs
- rsa.cs
- CustomValidator.cs
- PieceNameHelper.cs
- _ProxyChain.cs
- HttpCacheVaryByContentEncodings.cs
- ImageMap.cs
- PEFileEvidenceFactory.cs
- OutOfMemoryException.cs
- DbConnectionStringBuilder.cs
- SqlWebEventProvider.cs
- Normalization.cs
- Nodes.cs
- DataGridTable.cs
- LogPolicy.cs
- ProfileGroupSettingsCollection.cs
- WebPartActionVerb.cs
- ping.cs
- PlainXmlWriter.cs
- TextParagraphCache.cs
- WebBrowserBase.cs
- PersonalizableAttribute.cs
- Thumb.cs
- ValueTable.cs
- ParserStack.cs
- RepeatButtonAutomationPeer.cs
- ListBox.cs
- loginstatus.cs
- DataPagerFieldItem.cs