Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Interop / BrowserInteropHelper.cs / 1 / BrowserInteropHelper.cs
//---------------------------------------------------------------------------- // // File: BrowserInteropHelper.cs // // Description: Implements Avalon BrowserInteropHelper class, which helps // interop with the browser // // Created: 08/02/05 // // Copyright (C) by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Interop; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics; using MS.Internal; using MS.Internal.PresentationFramework; // SecurityHelper using MS.Win32; using System.Windows.Input; using MS.Internal.AppModel; namespace System.Windows.Interop { ////// Implements Avalon BrowserInteropHelper, which helps interop with the browser /// public static class BrowserInteropHelper { ////// Critical because it sets critical data. /// Safe because it is the static ctor, and the data doesn't go anywhere. /// [SecurityCritical, SecurityTreatAsSafe] static BrowserInteropHelper() { SetBrowserHosted(false); IsInitialViewerNavigation = true; } ////// Returns the IOleClientSite interface /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// Critical: Exposes a COM interface pointer to the IOleClientSite where the app is hosted /// PublicOK: It is public, but there is a demand /// public static object ClientSite { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); object oleClientSite = null; if (IsBrowserHosted) { Application.Current.BrowserCallbackServices.GetOleClientSite(out oleClientSite); } return oleClientSite; } } ////// Returns true if the app is a browser hosted app. /// public static bool IsBrowserHosted { get { return _isBrowserHosted.Value; } } ////// This is critical because setting the BrowserHosted status is a critical resource. /// [SecurityCritical] internal static void SetBrowserHosted(bool value) { _isBrowserHosted.Value = value; } ////// Returns the Uri used to launch the application. /// public static Uri Source { get { return _source.Value; } } ////// Sets the Source URI /// ////// Critical: critical because setting the source is critical. /// [SecurityCritical] internal static void SetSource(Uri source) { _source.Value = source; } ////// Returns true if we are running the XAML viewer pseudo-application (what used to be XamlViewer.xbap). /// This explicitly does not cover the case of XPS documents (MimeType.Document). /// internal static bool IsViewer { get { Application app = Application.Current; return app != null && app.MimeType == MimeType.Markup; } } ////// Returns true if avalon it top level. /// Also returns true if not browser-hosted. /// ////// Critical: Calls the SUCd IBrowserCallbackServices.IsAvalonTopLevel(). /// Safe: This is okay to dislose. /// internal static bool IsAvalonTopLevel { [SecurityCritical, SecurityTreatAsSafe] get { if (!IsBrowserHosted) return true; IBrowserCallbackServices bcs = Application.Current.BrowserCallbackServices; return bcs != null && bcs.IsAvalonTopLevel(); } } ////// Returns true if we are in viewer mode AND this is the first time that a viewer has been navigated. /// Including IsViewer is defense-in-depth in case somebody forgets to check IsViewer. There are other /// reasons why both IsViewer and IsViewerNavigation are necessary, however. /// ////// Critical: setting this information is a critical resource. /// internal static bool IsInitialViewerNavigation { get { return IsViewer && _isInitialViewerNavigation.Value; } [SecurityCritical] set { _isInitialViewerNavigation.Value = value; } } ////// Critical: this calls ForwardTranslateAccelerator, which is SUC'ed. /// [SecurityCritical] private static void HostFilterInput(ref MSG msg, ref bool handled) { // The host gets to see input, keyboard and mouse messages. if (msg.message == MS.Win32.NativeMethods.WM_INPUT || (msg.message >= MS.Win32.NativeMethods.WM_KEYFIRST && msg.message <= MS.Win32.NativeMethods.WM_IME_KEYLAST) || (msg.message >= MS.Win32.NativeMethods.WM_MOUSEFIRST && msg.message <= MS.Win32.NativeMethods.WM_MOUSELAST)) { if (MS.Win32.NativeMethods.S_OK == ForwardTranslateAccelerator(ref msg, false)) { handled = true; } } } ///This hook gets a "last chance" to handle a key. Such applicaton-unhandled /// keys are forwarded to the browser frame. /// ////// Critical: this calls ForwardTranslateAccelerator, which is SUC'ed. /// [SecurityCritical] internal static IntPtr PostFilterInput(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled) { if (!handled) { if (msg >= MS.Win32.NativeMethods.WM_KEYFIRST && msg <= MS.Win32.NativeMethods.WM_IME_KEYLAST) { MSG m = new MSG(hwnd, msg, wParam, lParam, SafeNativeMethods.GetMessageTime(), 0, 0); if (MS.Win32.NativeMethods.S_OK == ForwardTranslateAccelerator(ref m, true)) { handled = true; } } } return IntPtr.Zero; } ////// Critical: this attaches an event to ThreadFilterMessage, which requires an assert /// Safe: doesn't expose anything, just does some internal plumbing stuff /// [SecurityCritical, SecurityTreatAsSafe] internal static void InitializeHostFilterInput() { (new UIPermission(PermissionState.Unrestricted)).Assert(); // Blessed assert try { ComponentDispatcher.ThreadFilterMessage += new ThreadMessageEventHandler(HostFilterInput); } finally { UIPermission.RevertAssert(); } } private static SecurityCriticalDataForSet_isBrowserHosted; internal static SecurityCriticalDataForSet IsBrowserLowIntegrityProcess; private static SecurityCriticalDataForSet _isInitialViewerNavigation; private static SecurityCriticalDataForSet _source; /// /// Critical - call is SUC'ed /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport("PresentationHostDLL.dll", EntryPoint = "ForwardTranslateAccelerator")] private static extern int ForwardTranslateAccelerator(ref MSG pMsg, bool appUnhandled); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: BrowserInteropHelper.cs // // Description: Implements Avalon BrowserInteropHelper class, which helps // interop with the browser // // Created: 08/02/05 // // Copyright (C) by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Interop; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics; using MS.Internal; using MS.Internal.PresentationFramework; // SecurityHelper using MS.Win32; using System.Windows.Input; using MS.Internal.AppModel; namespace System.Windows.Interop { ////// Implements Avalon BrowserInteropHelper, which helps interop with the browser /// public static class BrowserInteropHelper { ////// Critical because it sets critical data. /// Safe because it is the static ctor, and the data doesn't go anywhere. /// [SecurityCritical, SecurityTreatAsSafe] static BrowserInteropHelper() { SetBrowserHosted(false); IsInitialViewerNavigation = true; } ////// Returns the IOleClientSite interface /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// Critical: Exposes a COM interface pointer to the IOleClientSite where the app is hosted /// PublicOK: It is public, but there is a demand /// public static object ClientSite { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); object oleClientSite = null; if (IsBrowserHosted) { Application.Current.BrowserCallbackServices.GetOleClientSite(out oleClientSite); } return oleClientSite; } } ////// Returns true if the app is a browser hosted app. /// public static bool IsBrowserHosted { get { return _isBrowserHosted.Value; } } ////// This is critical because setting the BrowserHosted status is a critical resource. /// [SecurityCritical] internal static void SetBrowserHosted(bool value) { _isBrowserHosted.Value = value; } ////// Returns the Uri used to launch the application. /// public static Uri Source { get { return _source.Value; } } ////// Sets the Source URI /// ////// Critical: critical because setting the source is critical. /// [SecurityCritical] internal static void SetSource(Uri source) { _source.Value = source; } ////// Returns true if we are running the XAML viewer pseudo-application (what used to be XamlViewer.xbap). /// This explicitly does not cover the case of XPS documents (MimeType.Document). /// internal static bool IsViewer { get { Application app = Application.Current; return app != null && app.MimeType == MimeType.Markup; } } ////// Returns true if avalon it top level. /// Also returns true if not browser-hosted. /// ////// Critical: Calls the SUCd IBrowserCallbackServices.IsAvalonTopLevel(). /// Safe: This is okay to dislose. /// internal static bool IsAvalonTopLevel { [SecurityCritical, SecurityTreatAsSafe] get { if (!IsBrowserHosted) return true; IBrowserCallbackServices bcs = Application.Current.BrowserCallbackServices; return bcs != null && bcs.IsAvalonTopLevel(); } } ////// Returns true if we are in viewer mode AND this is the first time that a viewer has been navigated. /// Including IsViewer is defense-in-depth in case somebody forgets to check IsViewer. There are other /// reasons why both IsViewer and IsViewerNavigation are necessary, however. /// ////// Critical: setting this information is a critical resource. /// internal static bool IsInitialViewerNavigation { get { return IsViewer && _isInitialViewerNavigation.Value; } [SecurityCritical] set { _isInitialViewerNavigation.Value = value; } } ////// Critical: this calls ForwardTranslateAccelerator, which is SUC'ed. /// [SecurityCritical] private static void HostFilterInput(ref MSG msg, ref bool handled) { // The host gets to see input, keyboard and mouse messages. if (msg.message == MS.Win32.NativeMethods.WM_INPUT || (msg.message >= MS.Win32.NativeMethods.WM_KEYFIRST && msg.message <= MS.Win32.NativeMethods.WM_IME_KEYLAST) || (msg.message >= MS.Win32.NativeMethods.WM_MOUSEFIRST && msg.message <= MS.Win32.NativeMethods.WM_MOUSELAST)) { if (MS.Win32.NativeMethods.S_OK == ForwardTranslateAccelerator(ref msg, false)) { handled = true; } } } ///This hook gets a "last chance" to handle a key. Such applicaton-unhandled /// keys are forwarded to the browser frame. /// ////// Critical: this calls ForwardTranslateAccelerator, which is SUC'ed. /// [SecurityCritical] internal static IntPtr PostFilterInput(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled) { if (!handled) { if (msg >= MS.Win32.NativeMethods.WM_KEYFIRST && msg <= MS.Win32.NativeMethods.WM_IME_KEYLAST) { MSG m = new MSG(hwnd, msg, wParam, lParam, SafeNativeMethods.GetMessageTime(), 0, 0); if (MS.Win32.NativeMethods.S_OK == ForwardTranslateAccelerator(ref m, true)) { handled = true; } } } return IntPtr.Zero; } ////// Critical: this attaches an event to ThreadFilterMessage, which requires an assert /// Safe: doesn't expose anything, just does some internal plumbing stuff /// [SecurityCritical, SecurityTreatAsSafe] internal static void InitializeHostFilterInput() { (new UIPermission(PermissionState.Unrestricted)).Assert(); // Blessed assert try { ComponentDispatcher.ThreadFilterMessage += new ThreadMessageEventHandler(HostFilterInput); } finally { UIPermission.RevertAssert(); } } private static SecurityCriticalDataForSet_isBrowserHosted; internal static SecurityCriticalDataForSet IsBrowserLowIntegrityProcess; private static SecurityCriticalDataForSet _isInitialViewerNavigation; private static SecurityCriticalDataForSet _source; /// /// Critical - call is SUC'ed /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport("PresentationHostDLL.dll", EntryPoint = "ForwardTranslateAccelerator")] private static extern int ForwardTranslateAccelerator(ref MSG pMsg, bool appUnhandled); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
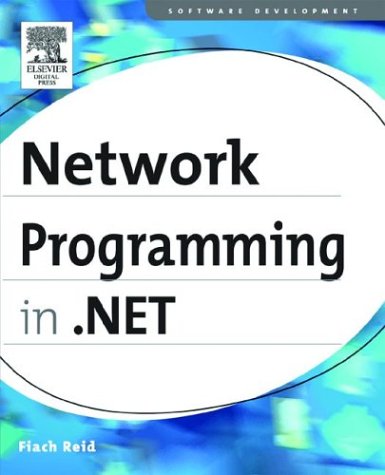
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlReader.cs
- OpenFileDialog.cs
- PrePostDescendentsWalker.cs
- ElementInit.cs
- DynamicActionMessageFilter.cs
- WindowClosedEventArgs.cs
- NavigatorOutput.cs
- RuntimeTrackingProfile.cs
- HtmlMeta.cs
- DocumentPropertiesDialog.cs
- cookie.cs
- DataRowCollection.cs
- TextBox.cs
- MimeBasePart.cs
- HostAdapter.cs
- Matrix.cs
- ImagingCache.cs
- _OverlappedAsyncResult.cs
- AssemblyInfo.cs
- SafePipeHandle.cs
- UndoManager.cs
- FormViewDeleteEventArgs.cs
- MarginsConverter.cs
- PropertyTabChangedEvent.cs
- TextSerializer.cs
- CodeRegionDirective.cs
- DataServiceExpressionVisitor.cs
- NamespaceExpr.cs
- ISAPIWorkerRequest.cs
- SqlSupersetValidator.cs
- ListControl.cs
- XmlByteStreamReader.cs
- XamlWrappingReader.cs
- BaseParaClient.cs
- SqlDependencyUtils.cs
- ToolStripOverflowButton.cs
- NativeMethods.cs
- OrderedDictionaryStateHelper.cs
- WebPartZoneDesigner.cs
- DispatcherOperation.cs
- Wildcard.cs
- LoginAutoFormat.cs
- DurationConverter.cs
- FastPropertyAccessor.cs
- FaultReasonText.cs
- InternalMappingException.cs
- AnnotationHelper.cs
- StringAttributeCollection.cs
- XmlAttributeOverrides.cs
- EventItfInfo.cs
- EventProviderWriter.cs
- ProfessionalColorTable.cs
- DeclaredTypeElement.cs
- ScrollBarRenderer.cs
- log.cs
- ZipIOExtraFieldElement.cs
- MediaPlayerState.cs
- NullReferenceException.cs
- _NegotiateClient.cs
- Models.cs
- MailDefinition.cs
- Command.cs
- ComponentManagerBroker.cs
- SqlUtils.cs
- Bind.cs
- EventLogStatus.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- _LocalDataStore.cs
- MappingModelBuildProvider.cs
- CapiSymmetricAlgorithm.cs
- ComponentManagerBroker.cs
- GridItemPattern.cs
- RadialGradientBrush.cs
- TraceLevelStore.cs
- SearchForVirtualItemEventArgs.cs
- ArrayConverter.cs
- ProtocolsSection.cs
- StreamAsIStream.cs
- RequestUriProcessor.cs
- DurationConverter.cs
- BufferedGraphicsContext.cs
- TreeWalkHelper.cs
- GroupStyle.cs
- SerializerProvider.cs
- SQLDoubleStorage.cs
- TreeViewCancelEvent.cs
- PenThreadPool.cs
- XmlProcessingInstruction.cs
- EditorPartChrome.cs
- Pkcs7Recipient.cs
- ScriptControlManager.cs
- ClientOptions.cs
- CharKeyFrameCollection.cs
- XmlSchemaAnnotation.cs
- ClientRuntimeConfig.cs
- EventLogPermissionEntry.cs
- HotSpot.cs
- documentsequencetextcontainer.cs
- IPHostEntry.cs
- Matrix.cs