Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / InputScopeNameConverter.cs / 1 / InputScopeNameConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: class for input scope type-converter // // Please refer to the design specfication http://avalon/Cicero/Specifications/Stylable%20InputScope.mht // // History: // 1/20/2005 : yutakan - created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Windows.Input; using System.ComponentModel; using System.Globalization; using System.ComponentModel.Design.Serialization; namespace System.Windows.Input { ////// type-converter which performs type conversions for inputscope /// ///http://avalon/Cicero/Specifications/Stylable%20InputScope.mht public class InputScopeNameConverter : TypeConverter { ////// Returns whether this converter can convert an object of one type to InputScope type /// InputScopeConverter only supports string type to convert from /// /// /// The conversion context. /// /// /// The type to convert from. /// ////// True if conversion is possible, false otherwise. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { // We can only handle string. if (sourceType == typeof(string)) { return true; } return false; } ////// Returns whether this converter can convert the object to the specified type. /// InputScopeConverter only supports string type to convert to /// /// /// The conversion context. /// /// /// The type to convert to. /// ////// True if conversion is possible, false otherwise. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (typeof (string) == destinationType && null != context && null != context.Instance && context.Instance is InputScopeName) { return true; } return false; } ////// Converts the given value to InputScope type /// /// /// The conversion context. /// /// /// The current culture that applies to the conversion. /// /// /// The source object to convert from. /// ////// InputScope object with a specified scope name, otherwise InputScope with Default scope. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { string stringSource = source as string; InputScopeNameValue nameValue = InputScopeNameValue.Default; InputScopeName inputScopeName; if (null != stringSource) { stringSource = stringSource.Trim(); if (-1 != stringSource.LastIndexOf('.')) stringSource = stringSource.Substring(stringSource.LastIndexOf('.')+1); if (!stringSource.Equals(String.Empty)) { nameValue = (InputScopeNameValue)Enum.Parse(typeof(InputScopeNameValue), stringSource); } } inputScopeName = new InputScopeName(); inputScopeName.NameValue = nameValue; return inputScopeName; } ////// Converts the given value as InputScope object to the specified type. /// This converter only supports string type as a type to convert to. /// /// /// The conversion context. /// /// /// The current culture that applies to the conversion. /// /// /// The value to convert. /// /// /// The type to convert to. /// ////// A new object of the specified type (string) converted from the given InputScope object. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { InputScopeName inputScopeName = value as InputScopeName; if (null != destinationType && null != inputScopeName) { if (destinationType == typeof(string)) { return Enum.GetName(typeof(InputScopeNameValue), inputScopeName.NameValue); } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: class for input scope type-converter // // Please refer to the design specfication http://avalon/Cicero/Specifications/Stylable%20InputScope.mht // // History: // 1/20/2005 : yutakan - created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Windows.Input; using System.ComponentModel; using System.Globalization; using System.ComponentModel.Design.Serialization; namespace System.Windows.Input { ////// type-converter which performs type conversions for inputscope /// ///http://avalon/Cicero/Specifications/Stylable%20InputScope.mht public class InputScopeNameConverter : TypeConverter { ////// Returns whether this converter can convert an object of one type to InputScope type /// InputScopeConverter only supports string type to convert from /// /// /// The conversion context. /// /// /// The type to convert from. /// ////// True if conversion is possible, false otherwise. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { // We can only handle string. if (sourceType == typeof(string)) { return true; } return false; } ////// Returns whether this converter can convert the object to the specified type. /// InputScopeConverter only supports string type to convert to /// /// /// The conversion context. /// /// /// The type to convert to. /// ////// True if conversion is possible, false otherwise. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (typeof (string) == destinationType && null != context && null != context.Instance && context.Instance is InputScopeName) { return true; } return false; } ////// Converts the given value to InputScope type /// /// /// The conversion context. /// /// /// The current culture that applies to the conversion. /// /// /// The source object to convert from. /// ////// InputScope object with a specified scope name, otherwise InputScope with Default scope. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { string stringSource = source as string; InputScopeNameValue nameValue = InputScopeNameValue.Default; InputScopeName inputScopeName; if (null != stringSource) { stringSource = stringSource.Trim(); if (-1 != stringSource.LastIndexOf('.')) stringSource = stringSource.Substring(stringSource.LastIndexOf('.')+1); if (!stringSource.Equals(String.Empty)) { nameValue = (InputScopeNameValue)Enum.Parse(typeof(InputScopeNameValue), stringSource); } } inputScopeName = new InputScopeName(); inputScopeName.NameValue = nameValue; return inputScopeName; } ////// Converts the given value as InputScope object to the specified type. /// This converter only supports string type as a type to convert to. /// /// /// The conversion context. /// /// /// The current culture that applies to the conversion. /// /// /// The value to convert. /// /// /// The type to convert to. /// ////// A new object of the specified type (string) converted from the given InputScope object. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { InputScopeName inputScopeName = value as InputScopeName; if (null != destinationType && null != inputScopeName) { if (destinationType == typeof(string)) { return Enum.GetName(typeof(InputScopeNameValue), inputScopeName.NameValue); } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
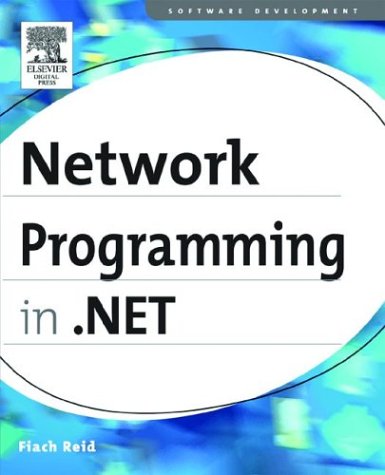
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataBinder.cs
- ConfigurationHelpers.cs
- DependentTransaction.cs
- clipboard.cs
- TextSchema.cs
- ComponentGuaranteesAttribute.cs
- PlanCompiler.cs
- ManagedIStream.cs
- IndentedWriter.cs
- PeerNameRegistration.cs
- Parallel.cs
- BinaryObjectWriter.cs
- HTMLTextWriter.cs
- CompilationSection.cs
- Rules.cs
- IResourceProvider.cs
- ExpressionBuilder.cs
- InvalidDataException.cs
- WebPartDeleteVerb.cs
- TypeConverterValueSerializer.cs
- GlyphCache.cs
- InternalSafeNativeMethods.cs
- Setter.cs
- MediaTimeline.cs
- FlowDocumentReader.cs
- EmptyStringExpandableObjectConverter.cs
- HttpResponseHeader.cs
- FunctionMappingTranslator.cs
- ProxyWebPartManager.cs
- XmlSchemaChoice.cs
- Util.cs
- GPPOINT.cs
- XmlAttributeHolder.cs
- ConfigurationHandlersInstallComponent.cs
- SqlDelegatedTransaction.cs
- DefaultIfEmptyQueryOperator.cs
- SmtpAuthenticationManager.cs
- IteratorFilter.cs
- Int32AnimationBase.cs
- FileDetails.cs
- ContentFileHelper.cs
- PersonalizationEntry.cs
- RegexTypeEditor.cs
- LinqDataSourceStatusEventArgs.cs
- WinEventQueueItem.cs
- Duration.cs
- ConfigXmlSignificantWhitespace.cs
- WebExceptionStatus.cs
- MatchingStyle.cs
- SchemaComplexType.cs
- TextFormatterImp.cs
- SymbolType.cs
- XhtmlBasicPhoneCallAdapter.cs
- login.cs
- RequestDescription.cs
- TypeSystem.cs
- IgnorePropertiesAttribute.cs
- ToRequest.cs
- ComNativeDescriptor.cs
- WebPartTracker.cs
- AppDomainProtocolHandler.cs
- CryptoSession.cs
- InvalidOperationException.cs
- Rule.cs
- PrincipalPermissionMode.cs
- NativeMethods.cs
- MemoryStream.cs
- DeclarativeCatalogPartDesigner.cs
- Context.cs
- LocatorBase.cs
- RotateTransform3D.cs
- LambdaExpression.cs
- UpDownBase.cs
- OleDbException.cs
- _TLSstream.cs
- WorkflowServiceAttributesTypeConverter.cs
- ArgumentsParser.cs
- DBNull.cs
- DataRelation.cs
- SecurityElementBase.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- DynamicRouteExpression.cs
- HtmlTextViewAdapter.cs
- StorageRoot.cs
- CircleHotSpot.cs
- AutomationInteropProvider.cs
- LinqDataSourceSelectEventArgs.cs
- WindowsComboBox.cs
- CalloutQueueItem.cs
- XPathNodeList.cs
- XsltException.cs
- WebPartEditVerb.cs
- BaseCAMarshaler.cs
- ADMembershipUser.cs
- Base64WriteStateInfo.cs
- XmlDigitalSignatureProcessor.cs
- ResXBuildProvider.cs
- VisualProxy.cs
- VolatileEnlistmentState.cs
- EmptyStringExpandableObjectConverter.cs